Webcam Capture in VB.NET Source code and Tutorial
Problem
Create a Windows Form Application program in Visual Basic.Net that will allow users to capture and save an image from a webcam.
Description
This tutorial will allow the user to select a video device, a video resolution and video input. Once set upped, the user can click the capture button to take an image from the video device or video input then the user can either save the image or reject it.
Before the tutorial the following are required to start:
- Microsoft Visual Studio 2008 – Above
- AForge.dll
- AForge.Video.DirectShow.dll
- AForge.Video.dll
The tutorial starts here:
- Download AForge.dll, AForge.Video.DirectShow.dll, AForge.Video.dll from the internet and save it to your preferred directory.
- Open Microsoft Visual Studio 2012
- Select a New Project on the File menu.
- Select Visual Basic, Windows Form Application then click OK.
- On the solution Panel, right click the “name of your project” then click add reference
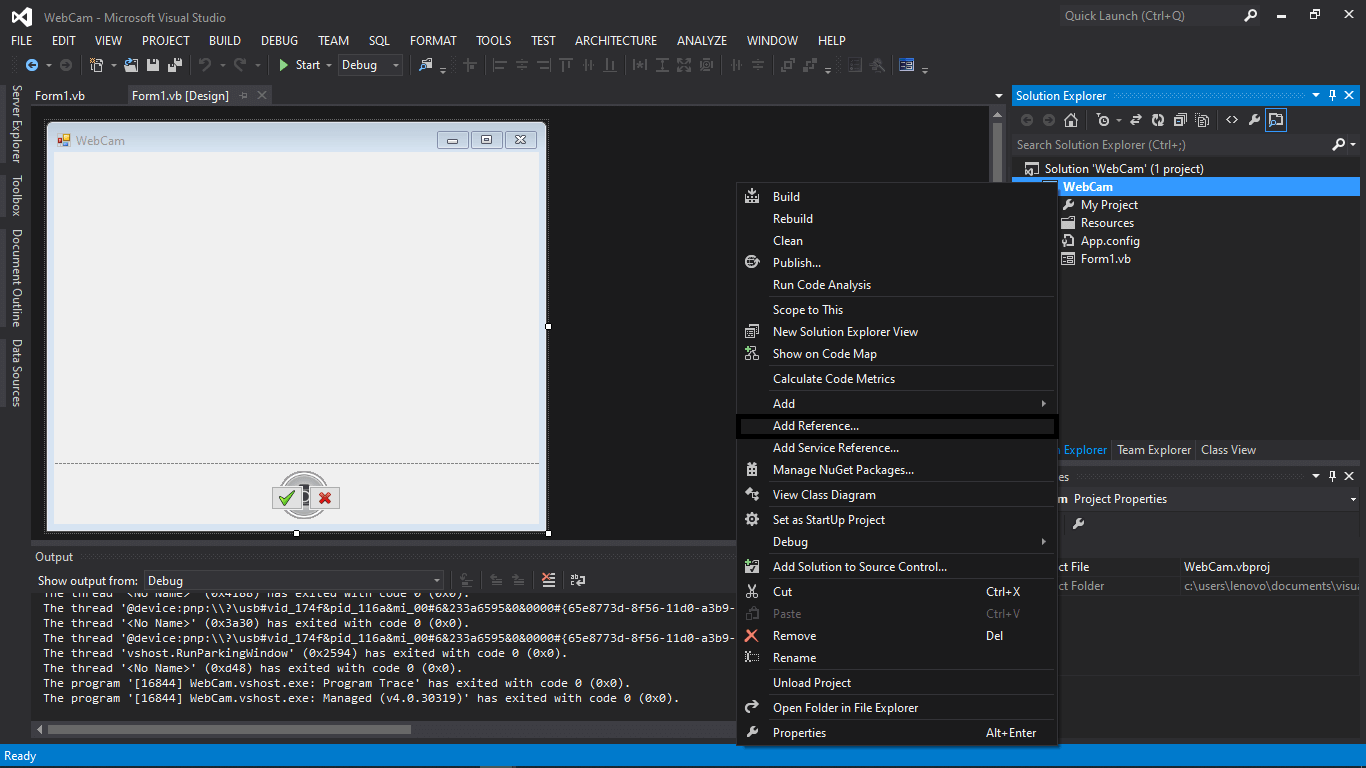
- On the reference manager, Click Browse on the left panel and check the MessagingToolkit.Barcode.dll, if it doesn’t appear, then click the Browse button on the bottom. Search and select for MessagingToolkit.Barcode.dll then click “Add”.
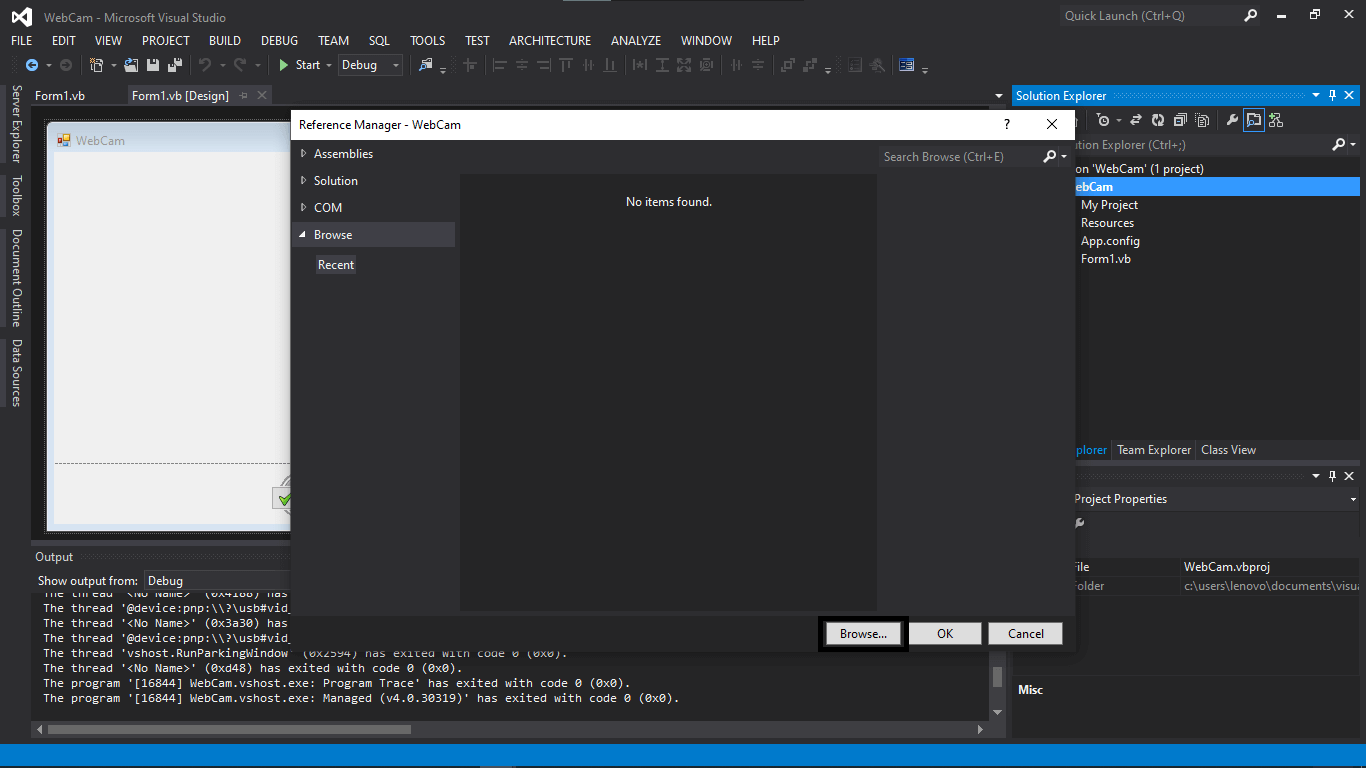
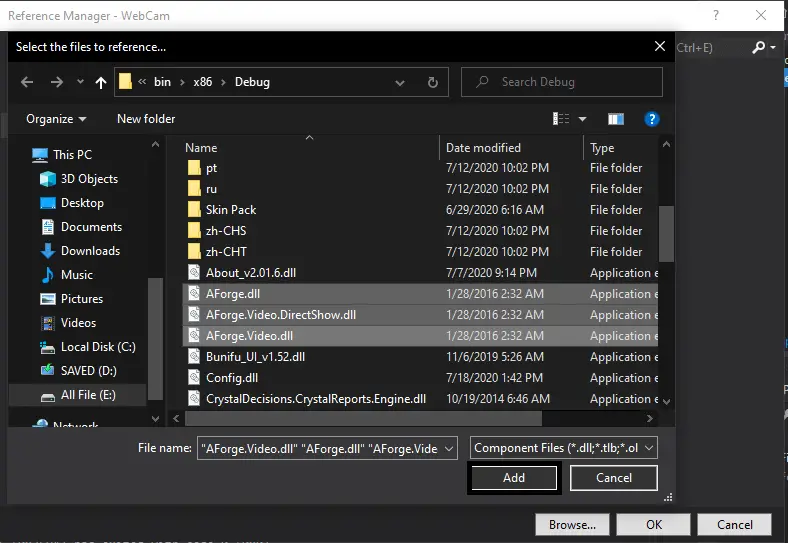
- Click “Ok” and we are ready now to design our form
- We need to design our form by the following controls:
- 3 Command Button – 1 button that can capture an image from the webcam, another button rejects the captured image and the last button that can save the captured image.
- 1 Picture Box – picture box where the captured image is displayed.
- We will also name our form controls in this way:
- cmdno is the name of the button for save.
- cmdok is the name of the button for save.
- pbcapture is the name of the button to generate the QR Code.
- PictureBox2 is the name of the picture box where the QR Code will be displayed.
- This is how we design the form. (Feel free to layout your own)
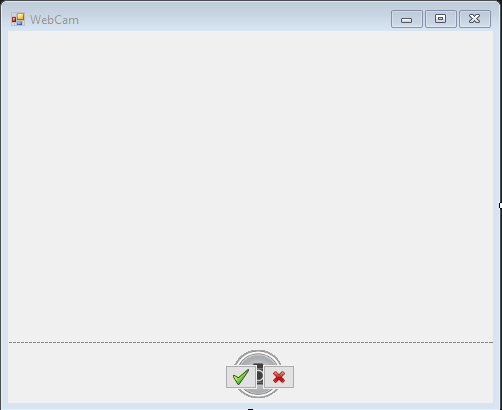
Figure 1. Design of the Form
- Import the .dll by pasting the code above the text editor
Code here
Imports AForge Imports AForge.Video Imports AForge.Video.DirectShow Imports System.IO
End Code
Code Explanation:
The codes above are needed, to enable the camera.
- below Public Class Form1, paste the following code:
Code here
Dim CAMARA As VideoCaptureDevice Dim BMP As Bitmap Dim Cap As String = "Capture"
End Code
Code Explanation:
The code will declare the variables above that to be used in the next series of codes.
- In the Form1_FormClosing event handler, paste the following codes
Code here
Try CAMARA.Stop() ' PictureBox2.Image = Nothing Catch ex As Exception Me.Dispose() 'PictureBox2.Image = Nothing End Try
End Code
Code Explanation:
The code will terminate the camera and return everything back to the initial state then closes the program.
- In the Form1_Load event handler, paste the following codes
Code here
cmdno.Visible = False cmdok.Visible = False Dim CAMARAS As VideoCaptureDeviceForm = New VideoCaptureDeviceForm() If CAMARAS.ShowDialog() = DialogResult.OK Then CAMARA = CAMARAS.VideoDevice AddHandler CAMARA.NewFrame, New NewFrameEventHandler(AddressOf CAPTURAR) CAMARA.Start() Else Me.Close() End If
End Code
Code Explanation:
The code will show a video capture device form dialogue, then once the user choses a video resolution, video device and video input and clicks “OK”, then it will proceed to the main form, else the form/program closes.
- Declare a Private Sub, naming it “CAPTURAR” then/or paste the following codes (it should be looked like below).
Code here
Private Sub CAPTURAR(sender As Object, eventArgs As NewFrameEventArgs) BMP = DirectCast(eventArgs.Frame.Clone(), Bitmap) pbcaptureimage.Image = DirectCast(eventArgs.Frame.Clone(), Bitmap) End Sub
End Code
Code Explanation:
This is the code for the capture button and also the code will capture a frame from the video device as a bitmap.
- Double click the pbcapture button then paste the following.
Code here
If Cap = "Capture" Then cmdno.Visible = True cmdok.Visible = True pbcapture.Visible = False CAMARA.Stop() Cap = "Start" ElseIf Cap = "Start" Then Dim CAMARAS As VideoCaptureDeviceForm = New VideoCaptureDeviceForm() CAMARA.Start() cmdno.Visible = False cmdok.Visible = False Cap = "Capture" End If End Sub
End Code
Code Explanation:
The code will display the captured image to the Picture box then it will hide the capture button and shows the check and ex button.
- Double click the x/no button then paste the following.
Code here
Dim CAMARAS As VideoCaptureDeviceForm = New VideoCaptureDeviceForm() CAMARA.Start() cmdno.Visible = False cmdok.Visible = False pbcapture.Visible = True Cap = "Capture"
End Code
Code Explanation:
The code will reject the captured image and re-initiate the camera. It will also hide the check/yes or ex/no button from the form and shows the capture button.
- Double click the check/yes button then paste the following.
Code here
Dim SD As New SaveFileDialog SD.InitialDirectory = My.Computer.FileSystem.SpecialDirectories.Desktop SD.FileName = "Visual Capture " & Date.Now.ToString("MM/dd/yy") SD.SupportMultiDottedExtensions = True SD.AddExtension = True SD.Filter = "PNG File|*.png" If SD.ShowDialog() = DialogResult.OK Then Try pbcapture image.Image.Save(SD.FileName, Imaging.ImageFormat.Png) Dim CAMARAS As VideoCaptureDeviceForm = New VideoCaptureDeviceForm() CAMARA.Start() cmdno.Visible = False cmdok.Visible = False pbcapture.Visible = True Cap = "Capture" Catch ex As Exception End Try Else Dim CAMARAS As VideoCaptureDeviceForm = New VideoCaptureDeviceForm() CAMARA.Start() cmdno.Visible = False cmdok.Visible = False pbcapture.Visible = True Cap = "Capture" End If
End Code
Code Explanation:
The code will save the captured image from the picture box into a special directory (the desktop) then It will hide the check/yes or ex/no button from the form and shows the capture button. It also re-initiates the camera.
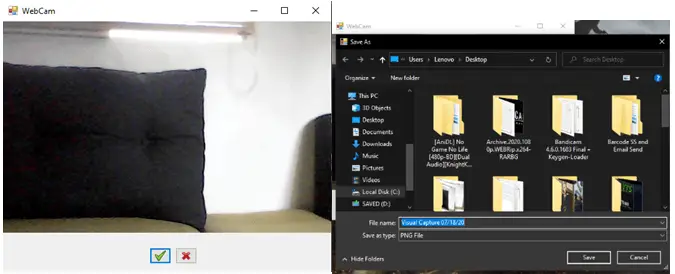
Author:
Name: Charlie Devera
Email Address: charliedevera000@gmail.com
Free Download Source code (WebCam Capture in VB.NET)
You may visit our facebook page for more information, inquiries and comments.
Hire our team to do the project.