Email Sender Code in VB.NET
Problem
Create a Windows Form Application program in Visual Basic.Net that will allow users to send Email
Description
This tutorial will allow the user to input the user/sender’s gmail account and password to send an email, with an attachment if desired, to the receiver’s gmail account.
Before the tutorial the following are required to start:
- Microsoft Visual Studio 2008 – Above
- Fast and Secure Internet
The tutorial starts here:
- Connect your device to the Internet.
- Open Microsoft Visual Studio 2012
- Select a New Project on the File menu.
- Select Visual Basic, Windows Form Application then click OK.
- We need to design our form by the following controls:
- 7 Labels – 6 labels for the Gmail Account, Password, Subject, Attachment, Receiver and Message text box, and 1 label to show Open File Dialogue.
- 6 Text box – text boxes for the Gmail Account, Password, Subject, Attachment, Receiver, and Message.
- 2 Command button – 1 button to send the message to the receiver and 1 button to cancel/close the form
- We will also name our form controls in this way:
- txtGmail is the name of the textbox for the Sender Gmail Account Text box.
- txtPassword is the name of the textbox for the Password Text box.
- txtSubject is the name of the textbox for the Subject Text box.
- txtattach is the name of the textbox for Attachment Text box.
- txtTo is the name of the textbox for the Receiver Gmail Account Text box.
- txtBody is the name of the textbox for the Message Text box.
- cmdSend is the name of the button for the Send Button.
- cmdCancel is the name of the button for the Cancel Button.
- lblBrowse is the name of the label to browse a file.
- This is how we design the form. (Feel free to layout your own)
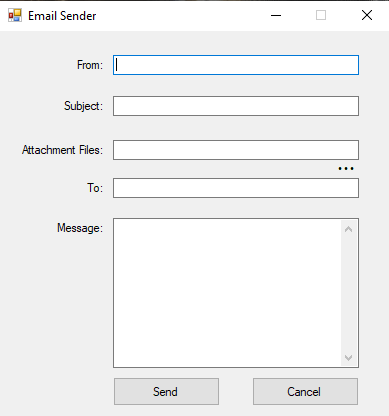
Figure 1. Design of the Form
- Double click the window form and paste the following code above the Form1_ load Handler.
Code here
Imports System.Net.Mail Public Class Form1 Dim Ofd As New OpenFileDialog End Code
Code Explanation:
The code above will enable email services and it also make the open file dialogue to be available once requested by the user.
- Double click the browse label and paste the following code.
Code here
Private Sub lblbrowse_Click(sender As Object, e As EventArgs) Handles lblbrowse.Click Ofd.Filter = "Image Files(*.Png,*.Jpg,*.Gif,*.SVG,*.Ico)|*.png;*.Jpg;*.Gif;*.SVG;*.Ico|Executetables(*.exe)|*.exe|Winzip(*.zip,*.7zip,*.rar)|*.zip;*.7zip;*.rar|Documents(*.mdb;*doc;*.xlsx,*.pptx,*.pub,*.sql,*.mdf)|*.mdb;*.doc;*.xlsx;*.pptx;*.pub;*.sql;*.mdf|Text File(*.txt,*.inf,*.ini)|*.txt;*.inf;*.ini|Dll Files(*.dll,*.sys,*.db,*.cfg)|*.dll;*.sys;*.db;*.cfg|Crystal Report(*.rpt)|*.rpt|All Files(*.*)|*.*" Ofd.FileName = "" If Ofd.ShowDialog() = Windows.Forms.DialogResult.OK Then txtattach.Text = Ofd.FileName End If End Sub
End Code
Code Explanation:
It will show an open file dialogue which support some file extensions,
- Double click the txtattach and paste the following code below the txtattach_TextChanged handler.
Code here
Private Sub txtattach_DragEnter(sender As Object, e As DragEventArgs) Handles txtattach.DragEnter If e.Data.GetDataPresent(DataFormats.FileDrop) Then e.Effect = DragDropEffects.Copy End If End Sub End Code
Code Explanation:
This will enable the user to drag and drop a file to the Attachment field.
- Double click the Cancel Button and paste the following code.
Code here
Private Sub cmdCancel_Click(sender As Object, e As EventArgs) Handles cmdCancel.Click Me.Dispose() End Sub
End Code
Code Explanation:
The code above will clear all the contents and exit the form.
- Double click the Send Button and paste the following code.
Code here
Private Sub btnsend_Click(sender As Object, e As EventArgs) Handles btnsend.Click If txtto.Text = "" Then MsgBox("Required Gmail...") ElseIf Not txtto.Text.EndsWith("@gmail.com") Then MsgBox("Invalid Format Gmail Account.", MsgBoxStyle.Exclamation) txtto.Text = "" Else Try Dim smtp_server As New SmtpClient Dim e_mail As New MailMessage() Dim attachment As System.Net.Mail.Attachment smtp_server.UseDefaultCredentials = False smtp_server.Credentials = New Net.NetworkCredential((txtgmail.Text), (txtpassword.Text)) smtp_server.Port = 587 smtp_server.EnableSsl = True smtp_server.Host = "smtp.gmail.com" e_mail = New MailMessage() e_mail.From = New MailAddress(txtgmail.Text) e_mail.IsBodyHtml = True e_mail.To.Add(txtto.Text) e_mail.Subject = txtsubject.Text e_mail.Body = txtmessage.Text attachment = New System.Net.Mail.Attachment(txtattach.Text) e_mail.Attachments.Add(attachment) smtp_server.Send(e_mail) MsgBox("Email Has Been Sent!", MsgBoxStyle.Information) txtsubject.Clear() txtto.Clear() txtattach.Clear() txtmessage.Clear() txtgmail.Clear() txtpassword.Clear() Me.Dispose() Catch ex As Exception MsgBox("Please Connect to the Internet Connection!", MsgBoxStyle.Information) End Try End If End Sub
End Code
Code Explanation:
The code will get all the information on the text boxes, send the message contained in the txtBody text box to the gmail of the receiver contained at the txtTo text box. It can also indicate whether the device is connected to the internet or the internet speed is too slow.
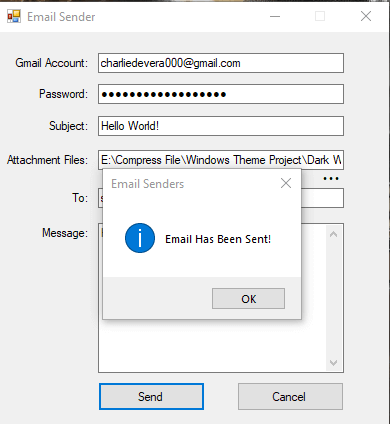
Conclusion:
With this tutorial and source code you can now send an email through this project. The project and source code is available for download and you may modify it based on your preferences and requirements.
Author:
Name: Charlie Devera
Email Address: charliedevera000@gmail.com
Free Download Source code (Email Sender in VB.Net)
You may visit our facebook page for more information, inquiries and comments.
Hire our team to do the project.