Basic Text Editor in VB.NET
This tutorial will guide you to a step by step tutorial on how to create a basic text editor in VB.Net
Problem
Create a Windows Form Application program in Visual Basic.Net that will allow users to make a basic text editor.
Description
This tutorial will allow the user to use different controls such as RichTextBox, ToolStrip, MenuStrip, StatusStrip, Timer, SaveFileDialog, OpenFileDialog to make a basic text editor in VB.NET.
Before the tutorial the following are required to start:
- Microsoft Visual Studio 2012 – Above
The tutorial starts here:
- Open Microsoft Visual Studio 2012
- Select a New Project on the File menu.
- Select Visual Basic, Windows Form Application then click OK.
- We need these following controls on our form.
- RichTextBox
- ToolStrip
- MenuStrip
- StatusStrip
- Timer
- SaveFileDialog
- OpenFileDialog
- Context Menu strip
- Add the items on the Menu bar, Tool Bar and Context Menu Strip first then we will name our form controls in this way:
- Document is the name for the Rich text box.
- Timer is the name for the timer.
- lblZoom is the name of a status strip label for zoom.
- lblCharCount is the name of another status strip for Character count.
- tbNew is the name of the tool bar item for New document.
- tbopen is the name of the tool bar item for Open document.
- tbsave is the name of the tool bar item for Save document.
- tbcut is the name of the tool bar item for Cut Text.
- tbcopy is the name of the tool bar item for Copy Text.
- tbpaste is the name of the tool bar item for Paste text.
- tbbold is the name of the tool bar item for Bold text.
- tbitalic is the name of the tool bar item for Italic text.
- tbunderline is the name of the tool bar item for Underline text.
- tbstrike is the name of the tool bar item for Strike text.
- tbalignleft is the name of the tool bar item for Align Left text.
- tbaligncentre is the name of the tool bar item for Align Center text.
- tbalignright is the name of the tool bar item for Align Right text.
- tbupper is the name of the tool bar item for Uppercase text.
- tblower is the name of the tool bar item for Lowercase text.
- tbzoom is the name of the tool bar item for Zoom In text.
- tbzoomout is the name of the tool bar item for Zoom out text.
- tbselectfont is the name of the tool bar item for text Font.
- tbselectsize is the name of the tool bar item for text size.
- mMNew is the name of the Main Menu item for New document.
- mMOpen is the name of the Main Menu item for Open document.
- mMSave is the name of the Main Menu item for Save document.
- mMExit is the name of the Main Menu item for Exit document.
- mMUndo is the name of the Main Menu item for Undo Text.
- mMRedo is the name of the Main Menu item for Redo Text.
- mMCut is the name of the Main Menu item for Cut Text.
- mMCopy is the name of the Main Menu item for Copy Text.
- mMPaste is the name of the Main Menu item for Paste Text.
- mMSelectAll is the name of the Main Menu item for Select All Text.
- mMCustomize is the name of the Main Menu item for Customize Text.
- UndoToolStripMenuItem is the name of the Context Menu item for Undo Text.
- RedoToolStripMenuItem is the name of the Context Menu item for Redo Text.
- CutToolStripMenuItem is the name of the Context Menu item for Cut Text.
- CopyToolStripMenuItem is the name of the Context Menu item for Copy Text.
- PasteToolStripMenuItem is the name of the Context Menu item for Paste Text.
- This is how we design the form. (Feel free to layout your own)
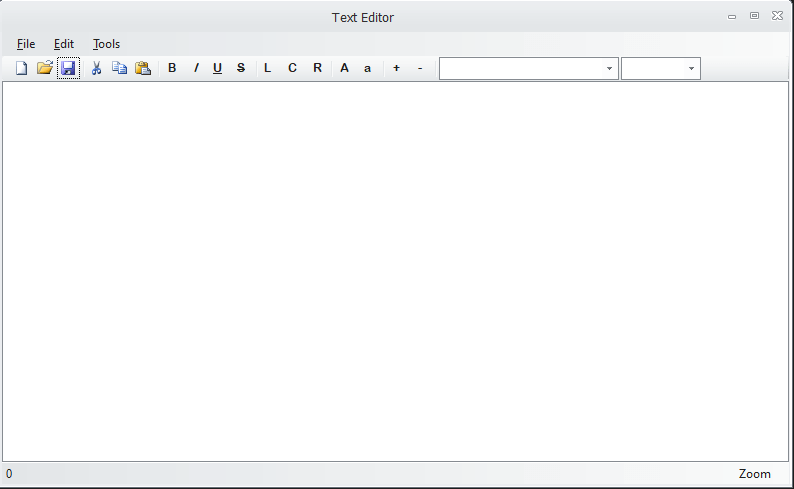
Figure 1. Design of the Form
- Paste the following code at the top of the text editor to import a service
Code here
Imports System.Drawing.Text
End Code
Code Explanation:
This will enable the editor to have access to different hotkeys related to text and to different functions and fonts installed in the device.
- Double click the window form and paste the following code:
Code here
For fntSize = 10 To 75 tbSelectSize.Items.Add(fntSize) Next Dim fonts As New InstalledFontCollection() For fntFamily As Integer = 0 To fonts.Families.Length - 1 tbSelectFont.Items.Add(fonts.Families(fntFamily).Name) Next
End Code
Code Explanation:
This code will load the font size and all the installed font and their font families to the text editor. This will also load the fonts in the Tbselectfont at the tool bar
- Double click the timer and paste the following code:
Code here
lblCharCount.Text = "Characters in the current document: " & Document.TextLength.ToString() lblZoom.Text = Document.ZoomFactor.ToString()
End Code
Code Explanation:
This code will count the number of characters and the zoom level in the text editor then displays it to lblCharCount label and lblZoom label.
- Double click the Rich Text box and paste the following code:
Code here
System.Diagnostics.Process.Start(e.LinkText)
End Code
Code Explanation:
This code starts a diagnostic process.
- For the code of Main Menu bar items, paste the following:
Code here
Private Sub mMNew_Click(sender As System.Object, e As System.EventArgs) Handles mMNew.Click NewDocument() End Sub Private Sub mMOpen_Click(sender As System.Object, e As System.EventArgs) Handles mMOpen.Click Open() End Sub Private Sub mMSave_Click(sender As System.Object, e As System.EventArgs) Handles mMSave.Click Save() End Sub Private Sub mMExit_Click(sender As System.Object, e As System.EventArgs) Handles mMExit.Click ExitApplication() End Sub '---------------------------- Private Sub mMUndo_Click(sender As System.Object, e As System.EventArgs) Handles mMUndo.Click Undo() End Sub Private Sub mMRedo_Click(sender As System.Object, e As System.EventArgs) Handles mMRedo.Click Redo() End Sub Private Sub mMCut_Click(sender As System.Object, e As System.EventArgs) Handles mMCut.Click Cut() End Sub Private Sub mMCopy_Click(sender As System.Object, e As System.EventArgs) Handles mMCopy.Click Copy() End Sub Private Sub mMPaste_Click(sender As System.Object, e As System.EventArgs) Handles mMPaste.Click Paste() End Sub Private Sub mMSelectAll_Click(sender As System.Object, e As System.EventArgs) Handles mMSelectAll.Click SelectAll() End Sub '----------------------- Private Sub mMCustomize_Click(sender As System.Object, e As System.EventArgs) Handles mMCustomize.Click Customize() End Sub
End Code
Code Explanation:
These codes will give a function for each item in the Menu bar/strip.
- For the toolbar items, paste the following code:
Code here
Private Sub tbNew_Click(sender As System.Object, e As System.EventArgs) Handles tbNew.Click NewDocument() End Sub Private Sub tbOpen_Click(sender As System.Object, e As System.EventArgs) Handles tbOpen.Click Open() End Sub Private Sub tbSave_Click(sender As System.Object, e As System.EventArgs) Handles tbSave.Click Save() End Sub Private Sub tbCut_Click(sender As System.Object, e As System.EventArgs) Handles tbCut.Click Cut() End Sub Private Sub tbCopy_Click(sender As System.Object, e As System.EventArgs) Handles tbCopy.Click Copy() End Sub Private Sub tbPaste_Click(sender As System.Object, e As System.EventArgs) Handles tbPaste.Click Paste() End Sub '--------------------------------- Private Sub tbBold_Click(sender As System.Object, e As System.EventArgs) Handles tbBold.Click Dim bfont As New Font(Document.Font, FontStyle.Bold) Dim rfont As New Font(Document.Font, FontStyle.Regular) If Document.SelectedText.Length = 0 Then Exit Sub If Document.SelectionFont.Bold Then Document.SelectionFont = rfont Else Document.SelectionFont = bfont End If End Sub Private Sub tbItalic_Click(sender As System.Object, e As System.EventArgs) Handles tbItalic.Click Dim Ifont As New Font(Document.Font, FontStyle.Italic) Dim rfont As New Font(Document.Font, FontStyle.Regular) If Document.SelectedText.Length = 0 Then Exit Sub If Document.SelectionFont.Italic Then Document.SelectionFont = rfont Else Document.SelectionFont = Ifont End If End Sub Private Sub tbUnderline_Click(sender As System.Object, e As System.EventArgs) Handles tbUnderline.Click Dim Ufont As New Font(Document.Font, FontStyle.Underline) Dim rfont As New Font(Document.Font, FontStyle.Regular) If Document.SelectedText.Length = 0 Then Exit Sub If Document.SelectionFont.Underline Then Document.SelectionFont = rfont Else Document.SelectionFont = Ufont End If End Sub Private Sub tbStrike_Click(sender As System.Object, e As System.EventArgs) Handles tbStrike.Click Dim Sfont As New Font(Document.Font, FontStyle.Strikeout) Dim rfont As New Font(Document.Font, FontStyle.Regular) If Document.SelectedText.Length = 0 Then Exit Sub If Document.SelectionFont.Strikeout Then Document.SelectionFont = rfont Else Document.SelectionFont = Sfont End If End Sub ' ------------------------------- Private Sub tbAlignLeft_Click(sender As System.Object, e As System.EventArgs) Handles tbAlignLeft.Click Document.SelectionAlignment = HorizontalAlignment.Left End Sub Private Sub tbAlignCentre_Click(sender As System.Object, e As System.EventArgs) Handles tbAlignCentre.Click Document.SelectionAlignment = HorizontalAlignment.Center End Sub Private Sub tbAlignRight_Click(sender As System.Object, e As System.EventArgs) Handles tbAlignRight.Click Document.SelectionAlignment = HorizontalAlignment.Right End Sub '---------------------------------------------- Private Sub tbUpper_Click(sender As System.Object, e As System.EventArgs) Handles tbUpper.Click Document.SelectedText = Document.SelectedText.ToUpper() End Sub Private Sub tbLower_Click(sender As System.Object, e As System.EventArgs) Handles tbLower.Click Document.SelectedText = Document.SelectedText.ToLower() End Sub '--------------------------------------- Private Sub tbZoom_Click(sender As System.Object, e As System.EventArgs) Handles tbZoom.Click If Document.ZoomFactor = 63 Then Exit Sub Else Document.ZoomFactor = Document.ZoomFactor + 1 End If End Sub Private Sub tbZoomOut_Click(sender As System.Object, e As System.EventArgs) Handles tbZoomOut.Click If Document.ZoomFactor = 1 Then Exit Sub Else Document.ZoomFactor = Document.ZoomFactor - 1 End If End Sub '------------------------------------------ Private Sub tbSelectFont_SelectedIndexChanged(sender As System.Object, e As System.EventArgs) Handles tbSelectFont.SelectedIndexChanged Dim ComboFonts As System.Drawing.Font Try ComboFonts = Document.SelectionFont Document.SelectionFont = New System.Drawing.Font(tbSelectFont.Text, Document.SelectionFont.Size, Document.SelectionFont.Style) Catch ex As Exception MsgBox(ex.Message) End Try End Sub Private Sub tbSelectSize_SelectedIndexChanged(sender As System.Object, e As System.EventArgs) Handles tbSelectSize.SelectedIndexChanged Document.SelectionFont = New Font(tbSelectSize.SelectedItem.ToString, CInt(tbSelectSize.SelectedItem.ToString), Document.SelectionFont.Style) End Sub
Encode
Code Explanation:
This code will give a function for each tools/item in the toolbar according what each tool must do.
- For the functions in the text editor, paste the following
Code here
'CLEAR THE RICHTEXTBOX - MAKES NEW DOCUMENT Private Sub NewDocument() Document.Clear() End Sub 'OPEN WORK AS PLAIN TEXT FILE Private Sub Open() If openWork.ShowDialog = Windows.Forms.DialogResult.OK Then Document.LoadFile(openWork.FileName, _ RichTextBoxStreamType.PlainText) End If End Sub 'SAVE WORK AS PLAIN TEXT FILE Private Sub Save() If saveWork.ShowDialog = Windows.Forms.DialogResult.OK Then Try Document.SaveFile(saveWork.FileName, _ RichTextBoxStreamType.PlainText) Catch ex As Exception MessageBox.Show(ex.Message) End Try End If End Sub 'EXIT THE PROGRAM Private Sub ExitApplication() Me.Close() End Sub
End Code
Code Explanation:
These codes are to shorten the code for each function used for the basic text editor, such as opening and saving the text file, clearing and exit the program.
- For the function in some tools in the text editor, paste the following:
Code here
Private Sub Undo() Document.Undo() End Sub Private Sub Redo() Document.Redo() End Sub Private Sub Cut() Document.Cut() End Sub Private Sub Copy() Document.Copy() End Sub Private Sub Paste() Document.Paste() End Sub Private Sub SelectAll() Document.SelectAll() End Sub Private Sub Customize() Dim ColorPicker As New ColorDialog() ColorPicker.AllowFullOpen = True ColorPicker.FullOpen = True ColorPicker.AnyColor = True If ColorPicker.ShowDialog = Windows.Forms.DialogResult.OK Then mainMenu.BackColor = ColorPicker.Color Tools.BackColor = ColorPicker.Color Status.BackColor = ColorPicker.Color End If
End Code
Code Explanation:
These codes are used to enable the functions on some tools on the toolbar, such as undo, copy, paste, cut, select all, redo, and costumize.
- For the tool in the toolstrip, paste the following codes:
Code here
Private Sub UndoToolStripMenuItem_Click(sender As System.Object, e As System.EventArgs) Handles UndoToolStripMenuItem.Click Undo() End Sub Private Sub RedoToolStripMenuItem_Click(sender As System.Object, e As System.EventArgs) Handles RedoToolStripMenuItem.Click Redo() End Sub Private Sub CutToolStripMenuItem_Click(sender As System.Object, e As System.EventArgs) Handles CutToolStripMenuItem.Click Cut() End Sub Private Sub CopyToolStripMenuItem_Click(sender As System.Object, e As System.EventArgs) Handles CopyToolStripMenuItem.Click Copy() End Sub Private Sub PasteToolStripMenuItem_Click(sender As System.Object, e As System.EventArgs) Handles PasteToolStripMenuItem.Click Paste() End Sub
End Code
Code Explanation:
This will assign a function for each tool in the context menu strip when right clicked.
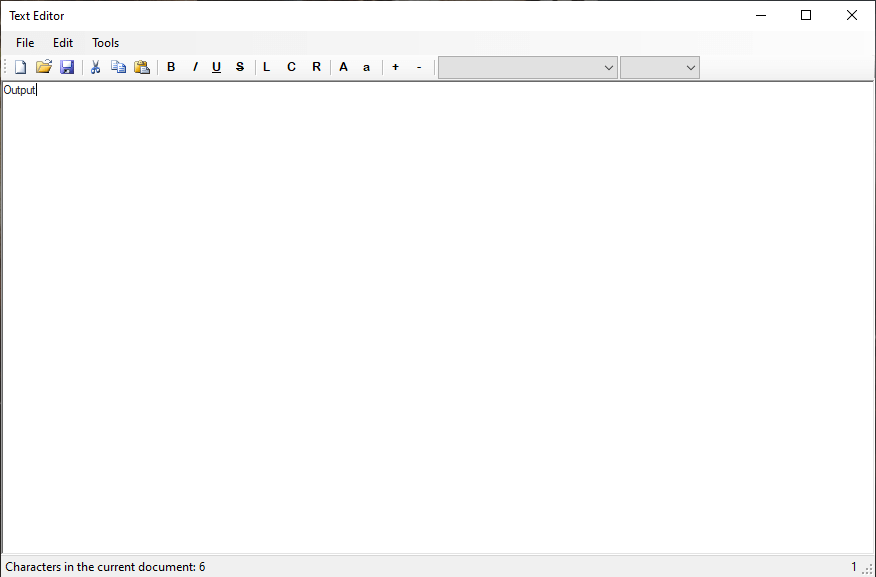
Conclusion:
With the use of text editor, you can now format your text and your content. The project and source code is available for download and you may modify it based on your preferences and requirements.
Author:
Name: Charlie Devera
Email Address: charliedevera000@gmail.com
Free Download Source code (Basic Text Editor in VB.Net)
You may visit our facebook page for more information, inquiries and comments.
Hire our team to do the project.