SMS Sender and Notification in VB.NET
Problem
Create a Windows Form Application program in Visual Basic.Net that will allow users to send SMS Notifications.
Description
This tutorial will allow the user to input their message in the text box and a receiver’s contact number to another text box. The message contained in the text box is sent to receiver’s contact number once the send button was clicked.
Before the tutorial the following are required to start:
- Microsoft Visual Studio 2008 – Above
- Broadband Stick/USB Modem
The tutorial starts here:
- Insert the Broadband Stick/USB Modem to the USB Port
- Open Microsoft Visual Studio 2012
- Select a New Project on the File menu.
- Select Visual Basic, Windows Form Application then click OK.
- We need to design our form by the following controls:
- 2 Labels – labels for the Phone Number Text box and Message text box
- 2 Text box – text boxes for the Phone Number and Message.
- 2 Command button – 1 button to send the message to the receiver and 1 button to cancel/close the form
- 2 Status strip label – label to show the status of the Port
- We will also name our form controls in this way:
- txtPhoneNumber is the name of the textbox for Phone Number
- txtMessage is the name of the textbox for Messages
- cmdSend is the name of the button for Send
- cmdCancel is the name of the button for Cancel
- ToolStripStatusLabel1 is the name of the first StatusStripLabel
- ToolStripStatusLabel2 is the name of the second StatusStripLabel
- This is how we design the form. (Feel free to layout your own)
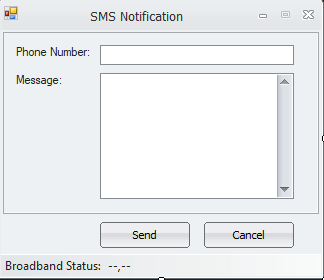
Figure 1. Design of the Form
- Double click the window form and paste the following code below.
Code here
Imports System.Management Imports System.Threading Imports System.IO.Ports Imports System.IO Public Class Form1 Public Function ModemsConnected() As String Dim modems As String = "" Try Dim searcher As New ManagementObjectSearcher( _ "root\CIMV2", _ "Select * from Win32_POTSModem") For Each queryobj As ManagementObject In searcher.Get() If queryobj("Status") = "OK" Then modems = modems & queryobj("AttachedTo") & "-" & queryobj("Description") & "***" End If Next Catch ex As Exception End Try Return modems End Function Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load Try Dim ports2() As String ports2 = Split(ModemsConnected(), "***") For i As Integer = 0 To ports2.Length - 2 Dim port_des As String = (ports2(i)) Dim gg As String = port_des gg = Trim(Mid(port_des, 1, 5)) Dim cleanstring As String = Replace(gg, "-", "") With SerialPort1 .PortName = cleanstring .BaudRate = 115200 .Parity = Parity.None .StopBits = StopBits.One .DataBits = 8 .Handshake = Handshake.RequestToSend .DtrEnable = True .RtsEnable = True .NewLine = vbCrLf .Open() ToolStripStatusLabel2.Text = "Connected." ToolStripStatusLabel2.ForeColor = Color.Green End With Next Catch ex As Exception ToolStripStatusLabel2.Text = "Disconnected." ToolStripStatusLabel2.ForeColor = Color.Red End Try End Sub
End Code
Code Explanation:
The code above will load and connect the connection from the broadband stick/USB Modem, and also can automatically update the Port for the connection between the device and the Broadband Stick/ USB Modem. It also change the appearance of the ToolStripStatusLabel2 to indicate if the device is connected.
- Double click the Cancel Button and paste the following code.
Code here
Private Sub cmdCancel_Click(sender As Object, e As EventArgs) Handles cmdCancel.Click Me.Dispose() End Sub
End Code
Code Explanation:
The code above will clear all the contents and exit the form.
- Double click the Send Button and paste the following code.
Code here
Private Sub cmdSend_Click(sender As Object, e As EventArgs) Handles cmdSend.Click Try If SerialPort1.IsOpen Then With SerialPort1 .Write("AT" & vbCrLf) .Write("AT+CMGF=1" & vbCrLf) .Write("AT+CMGS=" & Chr(34) & txtPhoneNumber.Text & Chr(34) & vbCrLf) .Write("[THIS IS SYSTEM GENERATED PLEASE DO NOT REPLY!]" & Environment.NewLine & txtMessage.Text & Chr(26)) End With Else MsgBox("Broadband is not Connected!", MsgBoxStyle.Exclamation, "Error") txtMessage.Focus() End If Catch ex As Exception txtMessage.Focus() End Try End Sub
End Code
Code Explanation:
The code will send the message contained in the txtMessage text box to the Contact Number contained at the txtPhoneNumber text box. It can also indicate whether the device is connected to the broadband stick/USB Modem.
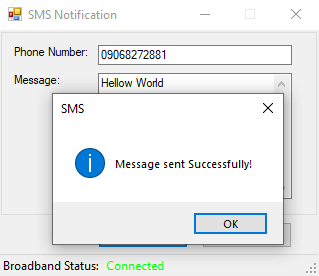
Conclusion:
This code snippets in vb.net will allow you to send a text to any phone number as long as the broadband has a load or balance. The application of this code can also be used to generate auto responses such as the grade inquiry and others. The project and source code is available for download and you may modify it based on your preferences and requirements.
Author:
Name: Charlie Devera
Email Address: charliedevera000@gmail.com
Free Download Source code (SMS Sender and Notification in VB.Net)
You may visit our facebook page for more information, inquiries and comments.
Hire our team to do the project.