Feet to Meter Converter in PHP Free Source code and Tutorial
Introduction
To create a feet to meter converter in PHP, you will need to create a function that takes in a value in feet and converts it to a value in meters. To do this, you will need to use the conversion factor of 0.3048. This means that 1 foot is equal to 0.3048 meters. To convert a value in feet to a value in meters, this article is for you.
This article will explain the source code on how to convert feet to meter in PHP. Let’s get started with the development.
Objectives
By the end of this tutorial, you will be able to:
- Create a front-end form in Bootstrap that allows the user to enter a value in feet.
- To create a PHP script that process the user input and convert the value enter in feet to meter.
- To integrate and apply the source code in your projects.
Relevant Source code
Requirements
- XAMPP
- Text editor (VS Code, Sublime, Brackets), download and install a text editor of your choice
Front-end
Line19-22 – this is the form used to capture the user input. The user will need to enter a number or value in feet. The form also includes the Convert button that will submit the user input to our back-end.

<form method="post"> <input type="text" name="measurement" class="form-control" placeholder="Input number in feet"><br/> <input type="submit" name="submit" class="btn btn-primary" value="Convert"> </form>
Back-end/PHP Script
Line 24-29 – this is the PHP script that will process the user input.
Line 26 – we have declared a variable $measureinfeet that will store the value entered by the user in the textbox.
Line 27 – this line will compute and display the conversion from feet to meter. To convert feet to meter we need to multiply the value entered in feet to 0.3048.

<?php if(isset($_POST['submit'])){ $measureinfeet = $_POST['measurement']; echo "<h4>".$measureinfeet." feet is equal to ". $measureinfeet*0.3048."M </h4>"; } ?>
Complete Source Code
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0, shrink-to-fit=no"> <title>Feet to Meter Converter in PHP</title> <link rel="stylesheet" href="assets/bootstrap/css/bootstrap.min.css"> </head> <body> <div class="row"> <div class="col-md-8 offset-md-2"> <div class="card"> <div class="card-header bg"> <h1>Feet to Meter Converter in PHP</h1> </div> <div class="card-body"> <form method="post"> <input type="text" name="measurement" class="form-control" placeholder="Input number in feet"><br/> <input type="submit" name="submit" class="btn btn-primary" value="Convert"> </form> <br> <?php if(isset($_POST['submit'])){ $measureinfeet = $_POST['measurement']; echo "<h4>".$measureinfeet." feet is equal to ". $measureinfeet*0.3048."M </h4>"; } ?> </div> </div> </div> </div> <script src="assets/js/jquery.min.js"></script> <script src="assets/bootstrap/js/bootstrap.min.js"></script> </body> </html>
Video Demo
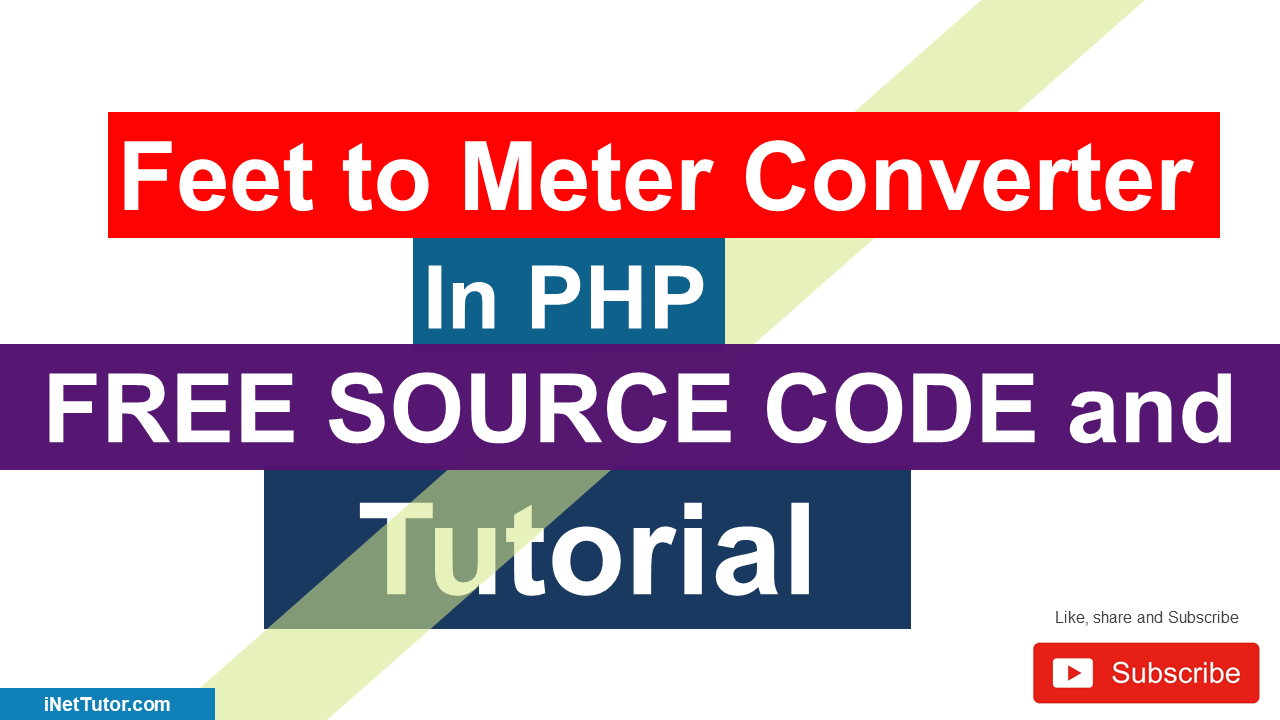
Summary
This tutorial covers the explanation of relevant source code used in creating a PHP script that will convert feet to meter. In addition, a form is also used to capture user input that sends the value to our PHP script for processing. For the complete reference please watch the video presentation on how to create a Feet to Meter converter in PHP.
We hope you found this tutorial to be helpful! Wishing you the best of luck with your projects! Happy Coding!
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.
Related Topics and Articles:
How to Generate Random Password in PHP Free Source code and Tutorial
Calculate Sum of Column in PHP and MySQL
Count Number of Characters in PHP Free Source code and Tutorial