Insert or Save Data into MySQL Database using C#
In this tutorial I will show you how to insert data in MySQL using C# in a simple way. Let’s follow tutorial below.
Step 1:
Create Project And Add Reference To C# WinForms Project. (visit the link to the first tutorial on how to add reference to our C# project)
How to connect MySQL Database to C# Tutorial and Source code
Step 2:
Open MySQL Workbench, right click and create schema (new database), give database name as “sampledb” and create table in database and give a name as “information”, then create columns id, emp_id, name, designation, username and password.

Step 3:
Back to the windows forms application and design information forms like this (see below picture)
- txtemp_id
- txtname
- txtdesignation
- txtusername
- txtpassword
- btnadd
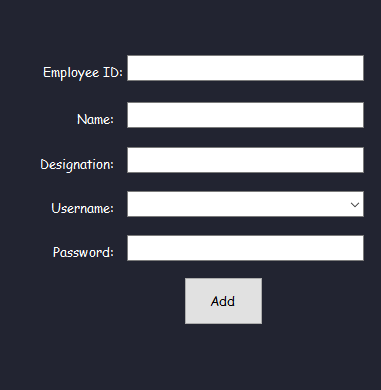
Step 4:
Source Code for Add button(btnadd) clicked event:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using MySql.Data.MySqlClient; namespace CSHARP_FULL_COURSE_WITH_MYSQL_DATABASE { public partial class information : Form { public information () { InitializeComponent(); } private void btnadd_Click(object sender, EventArgs e) { if (txtempid.Text == string.Empty || txtname.Text == string.Empty || txtdeisgnation.Text == string.Empty || txtusername.Text == string.Empty || txtpassword.Text == string.Empty) { MessageBox.Show("Empty Field", "VINSMOKE MJ", MessageBoxButtons.OK, MessageBoxIcon.Error); return; } else { MySqlConnection con = new MySqlConnection("datasource= localhost; database=sampledb;port=3306; username = root; password= db1234"); //open connection con.Open(); MySqlCommand cmd = new MySqlCommand("insert into information(emp_id,name,designation,username,password) values('" + txtempid.Text + "','" + txtname.Text + "','" + txtdeisgnation.Text + "','" + txtusername.Text + "','" + txtpassword.Text + "')",con); cmd.ExecuteNonQuery(); cmd.Dispose(); txtempid.Text = string.Empty; txtname.Text = string.Empty; txtdeisgnation.Text = string.Empty; txtusername.Text = string.Empty; txtpassword.Text = string.Empty; con.Close(); MessageBox.Show("Successfully Added", "VINSMOKE MJ", MessageBoxButtons.OK, MessageBoxIcon.Information); } } } }
Code Explanation:
This code serves as saving data or information in the database using this code will help you to understand the simple way to insert data, proper open and close connection.
Result:
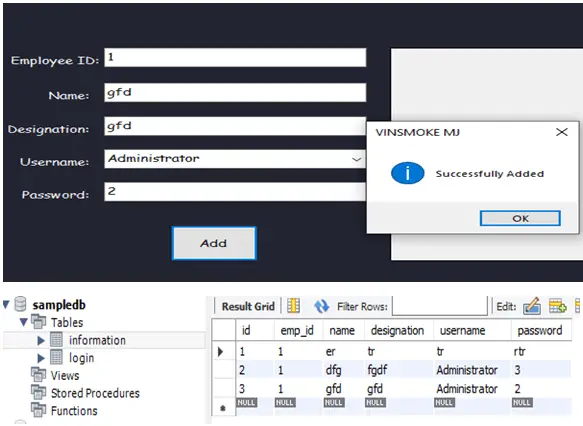
Insert or Save Data into MySQL Database using C# Free Download Source code
Mark Jaylo
https://www.youtube.com/watch?v=lgHGUeSUb8o&list=PLyrZdI7gZW7qhN-RwK1b5EqIzfHz5fh9F&index=3
You may visit our facebook page for more information, inquiries and comments.
Hire our team to do the project.