Square Root Calculator in C#
Introduction
Table of Contents
Finding the square root of a number is a fundamental mathematical operation that has wide-ranging applications in various fields. The square root of a number represents the value that, when multiplied by itself, yields the original number. It is denoted by the radical symbol (√) and is an essential concept in mathematics.
The importance of finding square roots extends beyond the realm of pure mathematics. In practical applications, square roots are used in calculations involving areas, distances, and measurements. For example, in geometry, finding the square root of the area allows us to determine the length of a side of a square or rectangle.
The objective of this lesson is to explore different methods to find the square root of a number in C#. By understanding the techniques and implementations, learners will gain the ability to perform square root calculations efficiently and accurately in their programs.
To achieve this, we will utilize the Math class in C#, which provides a wide range of mathematical functions and operations. The Math class simplifies the process of finding the square root by offering the sqrt function, which returns the square root of a given number.
Throughout this lesson, we will delve into the functionalities of the Math class, explore alternative methods for square root calculation, handle special cases, and provide practical examples to solidify the understanding of finding square roots in C#. Let’s dive into the world of square roots and unlock the power of mathematical operations in C#!
Objectives
Understanding the concept and methods for finding square roots in C# is essential for various programming tasks. This lesson aims to provide a comprehensive understanding of square roots, enabling learners to apply this knowledge effectively in their programs. Through exploration and practice, participants will learn to leverage C#’s Math class to compute square roots efficiently. By the end of the lesson, learners will be able to understand the underlying principles, learn the relevant syntax and methods, practice implementing square root calculations, and apply their newfound skills to solve real-world problems.
- Understand the concept of square roots and their significance in mathematics and programming.
- Learn the syntax and usage of C#’s Math class for computing square roots.
- Practice implementing square root calculations in C# programs through hands-on exercises.
- Apply the acquired knowledge to solve practical programming problems requiring square root calculations.
Source code example
using System; namespace SquareRootCalculator { class Program { static void Main() { Console.WriteLine("iNetTutor.com - Square Root Calculation"); // Prompt user to enter a number Console.Write("Enter a number to find its square root: "); string input = Console.ReadLine(); double number; if (!double.TryParse(input, out number)) { Console.WriteLine("Invalid input. Please enter a valid number."); return; } // Check if the number is non-negative if (number < 0) { Console.WriteLine("Cannot calculate square root of a negative number."); return; } // Calculate square root using Math.Sqrt method double squareRoot = Math.Sqrt(number); // Display the result Console.WriteLine($"Square root of {number} is: {squareRoot}"); // Check if the square root is a perfect square if (squareRoot % 1 == 0) { Console.WriteLine($"{squareRoot} is a perfect square."); } else { Console.WriteLine($"{squareRoot} is not a perfect square."); } Console.ReadLine(); } } }
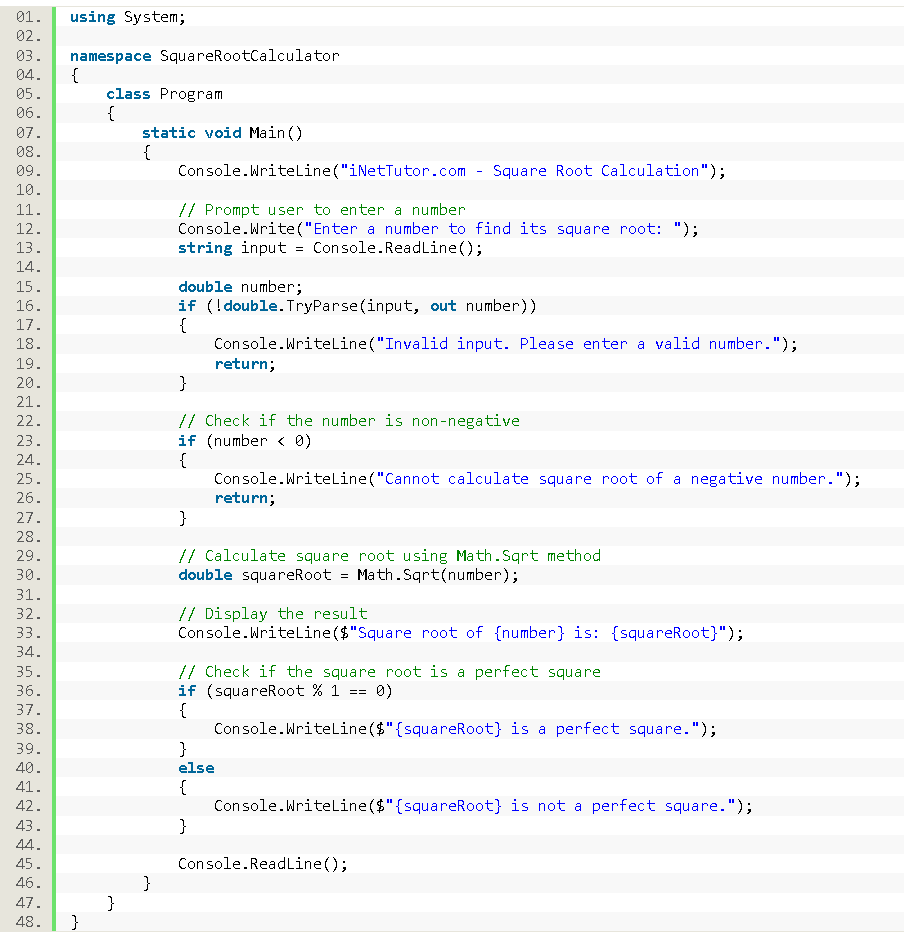
Explanation
The code provided is already well-written and includes error handling and additional functionality to check if the square root is a perfect square. Here’s a breakdown of the code:
- The code starts by displaying a message indicating the purpose of the program: “iNetTutor.com – Square Root Calculation”.
- The user is prompted to enter a number to find its square root.
- The double.TryParse() method is used to attempt parsing the user’s input. If the parsing fails (i.e., the input is not a valid number), an error message is displayed, and the program exits.
- The code checks if the entered number is negative. If it is, an error message is displayed, and the program exits.
- If the input is valid and non-negative, the Math.Sqrt() method is used to calculate the square root of the entered number.
- The calculated square root is displayed to the user.
- The code then checks if the square root is a perfect square by checking if it is divisible by 1 without a remainder. If it is, a message is displayed indicating that the square root is a perfect square. Otherwise, a message is displayed indicating that it is not a perfect square.
- Finally, the program waits for the user to press Enter before exiting.
Overall, the provided code is beginner-friendly, handles invalid input, calculates the square root, and provides additional functionality to check if the square root is a perfect square.
Output
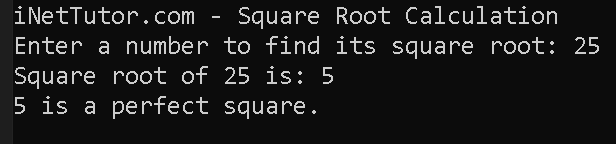
Summary
In this lesson, we explored the concept of square roots and learned how to find the square root of a number in C#. We started by understanding the mathematical representation of square roots and their significance in various applications, such as calculations involving areas and distances. The objective of the lesson was to find square roots of numbers in C# using the Math class. We discussed the functionalities of the Math class and its key method, Math.Sqrt(), which calculates the square root of a given number.
Throughout the lesson, we focused on four key objectives:
- Understanding the concept of square roots and their mathematical representation.
- Learning the techniques and methods for finding square roots in C# using the Math class and the Math.Sqrt() function.
- Practicing the implementation of square root calculations through coding exercises.
- Applying the knowledge of square roots to solve real-world problems in various domains.
We provided a beginner-friendly source code that demonstrated how to find the square root of a number in C# and included error trapping to handle invalid input. The code allowed users to enter a number and quickly find its square root, providing a solid starting point for understanding square root calculations in C#.
Key Points:
- Square roots represent the value that, when multiplied by itself, yields the original number.
- Finding square roots is important in various applications, such as calculations involving areas and distances.
- The Math class in C# provides a range of mathematical functions and operations.
- The Math.Sqrt() function is used to find the square root of a number in C#.
- Error trapping techniques can be used to handle invalid input and negative numbers.
- The double.TryParse() method can be used to validate user input.
- The Math class simplifies square root calculations by providing a ready-to-use function.
- The calculated square root can be displayed to the user for further use or analysis.
- Checking if the square root is a perfect square can provide additional insights into the result.
By understanding the concept of square roots, learning the techniques for finding square roots in C#, practicing through coding exercises, and applying the knowledge to real-world problems, learners have gained a solid foundation in square root calculations and can confidently utilize this mathematical operation in their C# programs.
Exercises and Assessment
To further improve the source code and provide a comprehensive learning experience, I suggest the following exercises, assessment, and lab exam:
Exercises:
- Error Handling Enhancement: Modify the source code to handle additional error scenarios, such as when the user enters non-numeric characters or when the input exceeds the range of double data type. Implement appropriate error messages and ensure the program gracefully handles these cases.
- Square Root Calculation Method: Create a separate method that takes a number as input and returns its square root. Refactor the source code to call this method for square root calculations instead of directly using Math.Sqrt(). This exercise will help reinforce the concept of methods and modular programming.
- Explain, in your own words, the steps involved in calculating the square root of a user-entered number using C#. Include how the program would handle an invalid input (e.g., a negative number).
Quiz
- Knowledge: Which of the following is used to convert user input from string to a numeric data type in C#?
a) Math class
b) double.TryParse()
c) Console.ReadLine()
d) Math.Sqrt() - Comprehension: What is the purpose of input validation in a program?
a) To ensure that the program executes without any errors
b) To convert user input to the desired data type
c) To validate the correctness and integrity of user input
d) To generate error messages for invalid input - Application: Which mathematical operator is used to calculate the remainder of a division operation in C#?
a) +
b) –
c) /
d) % - Analysis: In a program, if an error occurs during runtime, which part of the program is responsible for handling the error?
a) Compiler
b) IDE
c) Exception handler
d) Math class - Evaluation: If a program calculates the square root of a negative number, what should be the appropriate error message to display?
a) “Invalid input. Please enter a valid number.”
b) “Cannot calculate square root of a negative number.”
c) “Error: Invalid input. Please enter a positive number.”
d) “Error: Invalid input. Please enter a non-negative number.”
Related Topics and Articles:
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.