Admission System with Email Notification
This blog post will guide you through building a robust Admission System using PHP features like mysqli-prepared statements, Object-Oriented Programming (OOP), and Asynchronous JavaScript and XML (AJAX). This system will streamline the admission process for your organization, improve data security, and enhance user experience.
Introduction
Table of Contents
Managing admissions can be a paperwork nightmare for any organization, educational institution, or training program. Imagine sifting through mountains of applications, manually entering data, and chasing down missing information.
Traditional paper-based admission processes are:
- Time-consuming: Distributing, collecting, and sorting through paper applications is a laborious and lengthy process.
- Prone to Errors: Manual data entry increases the risk of errors and inconsistencies.
- Lacking Transparency: Applicants often have limited visibility into the application status.
- Inefficient Communication: Keeping applicants informed can be cumbersome.
There’s a better way! A web-based Admission System with email notification functionalities can revolutionize your intake process. Here’s how:
- Enhanced Efficiency: Applicants fill out applications online, eliminating paper and streamlining data collection.
- Improved Data Accuracy: Reduced manual entry minimizes errors and ensures data integrity.
- Real-Time Updates: Applicants can easily track their application status through online portals.
- Automated Communication: The system can automatically send email notifications to applicants upon submission, acknowledgement, or status updates.
- Streamlined Workflow: Admission staff can efficiently manage applications electronically, saving valuable time and resources.
By embracing a web-based Admission System, you can transform your intake process, improve communication with applicants, and free up valuable time to focus on what matters most – evaluating applications and selecting the best candidates.
Setting up the Development Environment
To set up a development environment for building a web-based Admission System, we’ll utilize XAMPP as our server stack, which includes Apache, MySQL, PHP, and Perl, and a text editor for writing code. Here are the steps to install XAMPP and a text editor of your choice:
- Installing XAMPP:
- Visit the official XAMPP website (https://www.apachefriends.org/index.html) and download the latest version of XAMPP for your operating system (Windows, macOS, or Linux).
- Run the installer and follow the on-screen instructions to complete the installation process.
- During the installation, you’ll be prompted to select the components you want to install. Ensure that Apache, MySQL, PHP, and phpMyAdmin are selected.
- Choose the installation directory (the default is usually fine) and complete the installation.
- Once XAMPP is installed, launch the control panel and start the Apache and MySQL services.
- Installing a Text Editor:
- Choose a text editor for writing code. Some popular options include Visual Studio Code, Sublime Text, Atom, and Notepad++.
- Visit the official website of your chosen text editor and download the installer for your operating system.
- Run the installer and follow the on-screen instructions to complete the installation process.
- Once installed, launch the text editor to begin writing code for your Admission System.
- Setting up the Necessary Software Components:
- With XAMPP running, open a web browser and navigate to http://localhost/phpmyadmin/. This will open phpMyAdmin, a web-based interface for managing MySQL databases.
- Log in to phpMyAdmin using the default username “root” and leave the password field blank (unless you set a password during XAMPP installation).
- Create a new database for the Admission System by clicking on the “Databases” tab, entering a name for the database (e.g., “admission_system”), and clicking “Create”.
- Now, you’re ready to start developing your Admission System. Create a new folder in the XAMPP “htdocs” directory (usually located at “C:\xampp\htdocs” on Windows or “/Applications/XAMPP/htdocs” on macOS) to store your project files.
- Testing the Environment:
- Create a new PHP file (e.g., test.php) in your project folder and open it in your text editor.
- Write a simple PHP script in the file, such as <?php phpinfo(); ?>.
- Save the file and access it through a web browser by navigating to http://localhost/test.php. You should see a PHP info page displaying information about your PHP configuration.
By following these steps, you can install XAMPP and a text editor of your choice to set up a development environment for building your web-based Admission System. This environment provides the necessary tools and infrastructure to develop, test, and debug your PHP application locally before deploying it to a live server.
Database Design
A well-designed database is the backbone of your Admission System. Here, we’ll discuss the structure and organization of the database tables to store admission-related data effectively.
Core Tables:
- Applicants: This table will store information about the individuals applying for admission. Here are some essential fields:
- applicant_id (INT, PRIMARY KEY): Unique identifier for each applicant.
- first_name (VARCHAR): Applicant’s first name.
- last_name (VARCHAR): Applicant’s last name.
- email (VARCHAR): Applicant’s email address (important for notifications).
- phone_number (VARCHAR): Applicant’s phone number (optional).
- other_details (TEXT): Optional field for storing additional applicant information (e.g., resume URL, portfolio link).
- Courses: This table will store details about the courses or programs offered:
- course_id (INT, PRIMARY KEY): Unique identifier for each course.
- course_name (VARCHAR): Name of the course or program.
- description (TEXT): Detailed description of the course curriculum and requirements.
- start_date (DATE): Date the course or program starts.
- end_date (DATE): Date the course or program ends (optional).
- Applications: This table will link applicants to the courses they are applying for:
- application_id (INT, PRIMARY KEY): Unique identifier for each application.
- applicant_id (INT, FOREIGN KEY references Applicants(applicant_id)): Connects the application to a specific applicant in the Applicants table.
- course_id (INT, FOREIGN KEY references Courses(course_id)): Connects the application to a specific course in the Courses table.
- application_date (DATETIME): Date and time the application was submitted.
- additional_documents (TEXT): Optional field for storing links to uploaded documents (e.g., transcripts, essays).
Additional Tables (Optional):
- Admission_Status: This table can be used to define different application status options (e.g., “Pending”, “Approved”, “Rejected”) and link them to applications in the Applications table with a foreign key relationship.
- User_Accounts: If you plan to implement user authentication for managing the admission process, you’ll need a separate table to store user credentials securely.
Relationships:
The Applicants, Courses, and Applications tables are linked together using foreign keys. An applicant can submit multiple applications for different courses (Applicants has a one-to-many relationship with Applications). Similarly, a course can have many applicants applying for it (Courses has a one-to-many relationship with Applications).
These are just a few examples of the tables and fields that may be required in the database design for an Admission System with Email Notification. The actual structure and organization of the database may vary depending on the specific needs and requirements of the educational institution.
It’s important to ensure that the tables are properly related through primary and foreign keys to establish the necessary connections between the different tables. This allows for efficient retrieval and manipulation of data during the admission process.
User Interface Design
The user interface (UI) is the face of your Admission System. It’s where applicants interact with the system to submit their applications. Here’s why a well-designed UI is crucial:
- Improved User Experience: An intuitive and user-friendly interface makes the application process smooth and frustration-free for applicants.
- Increased Application Completions: A clear and easy-to-follow UI encourages applicants to complete the application process without getting lost or discouraged.
- Enhanced Brand Image: A professional-looking UI reflects positively on your organization and builds trust with potential applicants.
Here’s how we can achieve this using HTML, CSS, and JavaScript:
- Designing the Applicant Interface (HTML):
- Use HTML to structure the web page layout, including clear sections for applicant information, course selection, application submission, and potentially a confirmation message.
- Create well-labeled form fields using HTML form elements like <input>, <textarea>, and <select>.
- Consider using clear and concise labels for each field to guide applicants through the process.
- Styling for Visual Appeal (CSS):
- Apply CSS to style the web page with a visually appealing and consistent design.
- Use CSS to format the layout, fonts, colors, and overall look and feel of the interface.
- Ensure the UI is responsive and adapts to different screen sizes (desktop, tablet, mobile) for optimal user experience.
- Enhancing Interactivity (JavaScript):
- Implement basic JavaScript for client-side validation of user input. This can include checking for missing required fields, ensuring email addresses are formatted correctly, or limiting the length of text entries.
- Consider using JavaScript for interactive elements like dropdown menus, accordion sections for displaying additional information, or progress bars to indicate how far along the applicant is in the process.
Usability Testing is Key:
- As you develop the UI, involve potential users (students, applicants) in usability testing. Gather feedback on the clarity, ease of use, and overall user experience of the interface.
- Based on the feedback, refine the UI to ensure it’s user-friendly and caters to the needs of your target audience.
Remember: Don’t overload the UI with unnecessary elements. Strive for a clean, well-organized interface that guides applicants efficiently through the admission process.
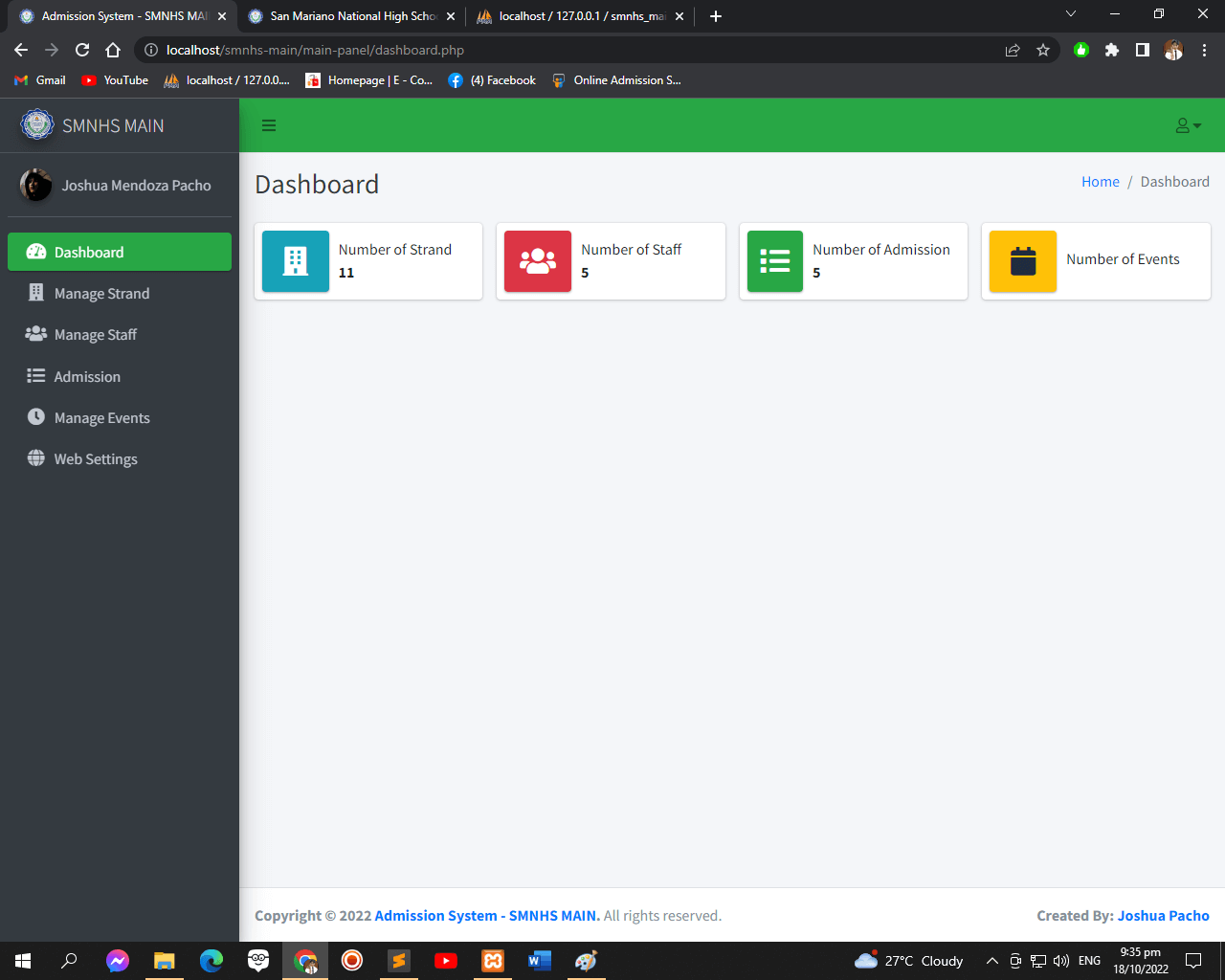
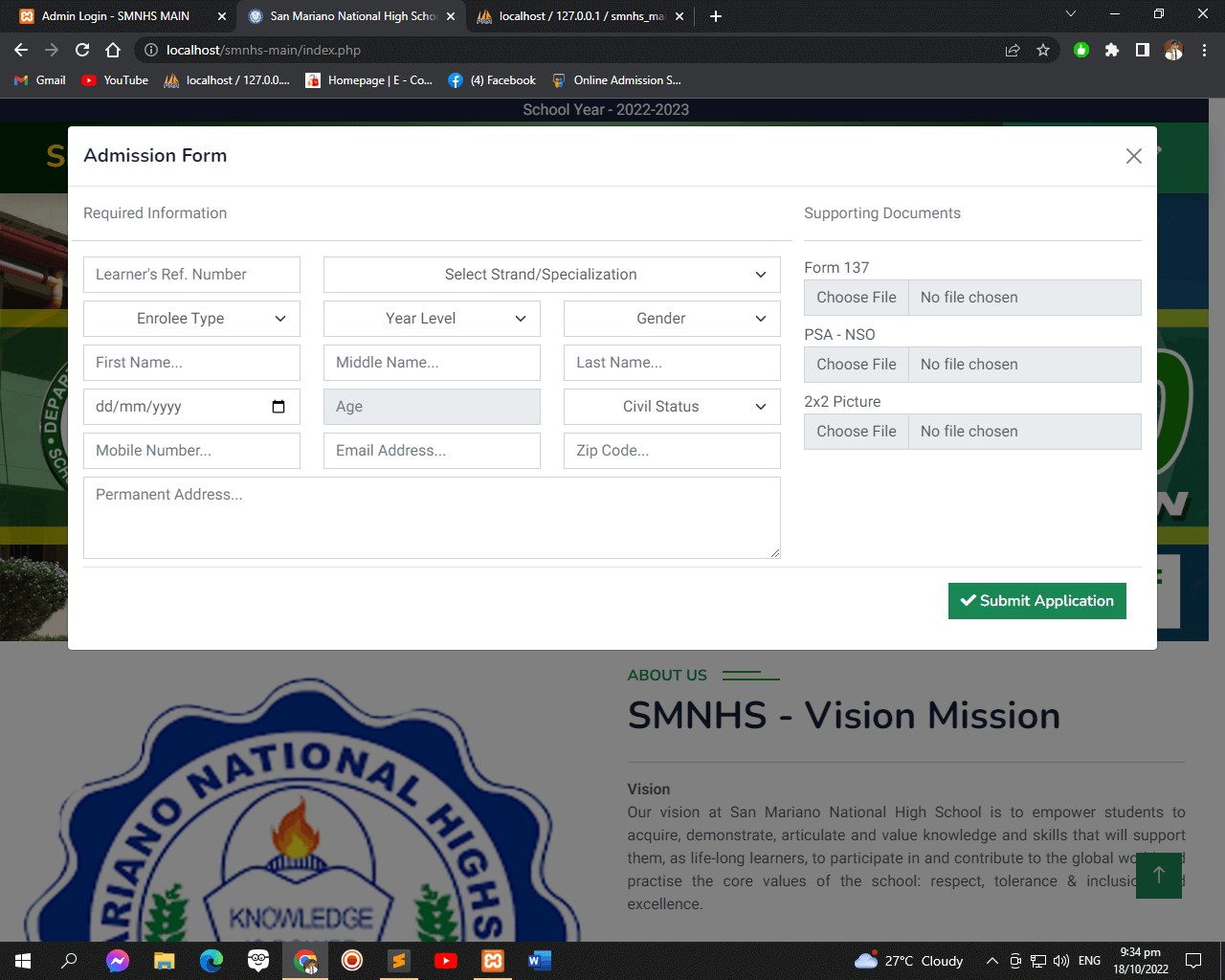
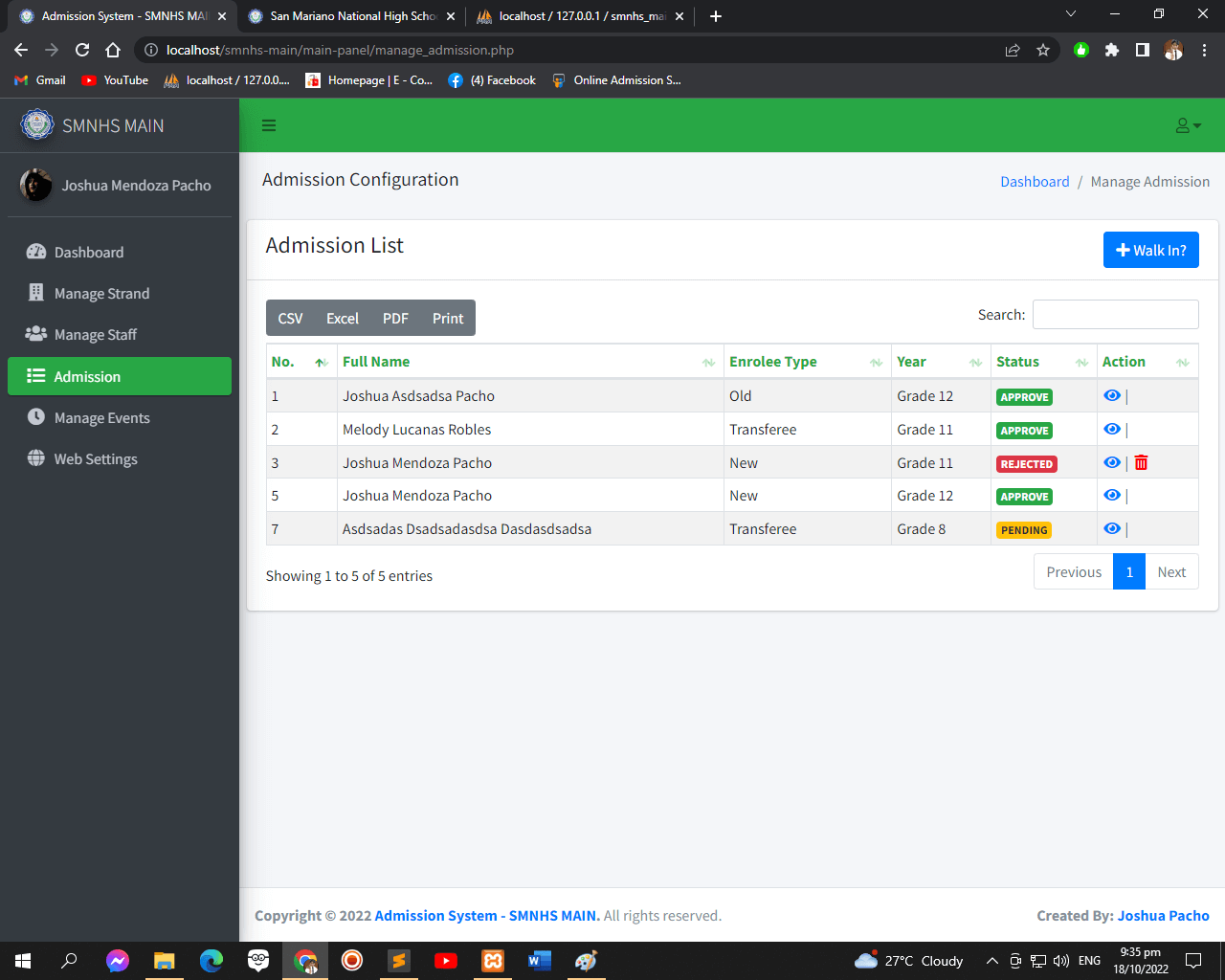
Implementing the Backend Functionality
In building the backend functionality for the Admission System, we’ll focus on secure database interactions using mysqli-prepared statements, implementing CRUD operations for admission-related data, and emphasizing the importance of validating user input to prevent security vulnerabilities.
- mysqli for Database Communication:
We’ll use the mysqli extension in PHP to connect to the MySQL database, execute queries, and retrieve data. However, security is paramount! Standard SQL queries are vulnerable to SQL injection attacks, where malicious users can inject code into your queries to manipulate or steal data.
Enter mysqli_prepared_statements:
- These statements provide a secure way to interact with the database.
- You define the SQL query with placeholders (?) for dynamic values.
- Later, you bind the actual user input data to these placeholders, ensuring separation between the query structure and the data itself.
Here’s a simplified example of how a secure insertion using a prepared statement might look:
<?php $stmt = $mysqli->prepare("INSERT INTO Applicants (first_name, last_name, email) VALUES (?, ?, ?)"); $stmt->bind_param("sss", $firstName, $lastName, $email); $stmt->execute(); $stmt->close(); ?>
- Steps involved in implementing CRUD operations for admission-related data:
- Create (INSERT): To add a new admission application or student record to the database, use an INSERT query with mysqli-prepared statements. Gather input from the user via a form, validate it, and then execute the prepared statement.
- Read (SELECT): To retrieve admission-related data from the database, use a SELECT query. Filter and retrieve specific records based on criteria such as student ID, course ID, or application status.
- Update (UPDATE): To modify existing admission records, use an UPDATE query. Gather input from the user via a form, validate it, and then execute the prepared statement to update the relevant record in the database.
- Delete (DELETE): To remove admission records from the database, use a DELETE query. Execute the query with appropriate conditions to delete specific records based on criteria such as student ID or application status.
- Importance of validating user input to prevent security vulnerabilities:
- Validating user input is critical for preventing security vulnerabilities such as SQL injection, XSS (Cross-Site Scripting), and CSRF (Cross-Site Request Forgery).
- Input validation ensures that data entered by users meets specific criteria (e.g., format, length, range) before it is processed or stored in the database.
- Use server-side validation to sanitize and validate user input before interacting with the database. This includes checking for empty fields, validating email addresses, and sanitizing input to prevent SQL injection attacks.
- Client-side validation using JavaScript can provide immediate feedback to users, but server-side validation is essential for security as client-side validation can be bypassed.
- Implementing input validation and sanitization reduces the risk of malicious users exploiting vulnerabilities in the application and helps maintain the integrity and security of the Admission System.
Remember: Security should be a top priority throughout your development process. Using mysqli prepared statements and validating user input are essential steps in building a robust and secure Admission System.
Implementing Email Notification
A key feature of your Admission System is keeping applicants informed about their application status. Here, we’ll explore integrating email functionality using PHP’s built-in mail function or a third-party library.
- Choosing Your Email Sending Method:
There are two main approaches:
- PHP mail function: This is a basic option included in PHP. It allows you to send emails directly from your web server. However, it can have limitations in terms of reliability and security.
- Third-party libraries: Popular options like PHPMailer or SwiftMailer offer a more robust solution. They provide features like email formatting (HTML emails), attachments, and integration with external email services (e.g., SendGrid) for improved deliverability and security.
- Sending Admission Status Emails:
Once you’ve chosen your email sending method, here’s the general process:
- Triggering the Email: The email notification can be triggered based on specific events in your Admission System. For example, upon successful application submission, an email confirming receipt can be sent. Similarly, emails can be sent for status updates (e.g., application approved, rejected).
- Constructing the Email: Create the email content dynamically using PHP. This might include:
- Applicant name and other relevant details.
- Specific message regarding the application status (e.g., “Congratulations! Your application has been approved”).
- Additional information or instructions (e.g., next steps in the admission process, login credentials for an applicant portal).
- Sending the Email: Use the chosen method (PHP mail function or third-party library) to send the email to the applicant’s email address stored in the database.
- Security Considerations:
- Never send passwords or sensitive information in plain text. Consider hashing or encrypting sensitive data before storing it in the database.
- Implement mechanisms to prevent email spoofing. This involves techniques to ensure the email appears to be from your legitimate organization and not a malicious source.
- Testing Thoroughly:
Before deploying your system, thoroughly test the email notification functionality. Send test emails to different email addresses to ensure they are delivered correctly and formatted as expected.
By integrating email notification, you can significantly enhance the user experience of your Admission System. Applicants will appreciate being kept informed about the status of their application, improving transparency and communication throughout the process.
Implementing AJAX for Dynamic Updates
Imagine an Admission System where applicants don’t have to refresh the entire page after submitting an application or checking their status. This is where AJAX (Asynchronous JavaScript and XML) comes into play.
What is AJAX?
AJAX allows for asynchronous communication between a web page and the server. This means that the web page can send requests to the server for data or processing without needing a full page reload. This leads to a more dynamic and responsive user experience.
Benefits of using AJAX in the Admission System:
- Improved User Experience: Applicants don’t have to wait for the entire page to reload, creating a smoother and faster experience.
- Real-time Updates: Data can be updated dynamically without full page refreshes. Imagine displaying a confirmation message after application submission or updating an application status indicator without reloading the entire page.
- Reduced Server Load: Only the necessary data is exchanged with the server, reducing overall server load compared to full page refreshes.
How can we use AJAX in our Admission System?
Here are some potential applications:
- Form Validation: Use AJAX to validate user input on the client-side (applicant’s web browser) before submitting the application form. This provides immediate feedback to the applicant, allowing them to correct errors without waiting for a server response.
- Dynamic Content Updates: Imagine scenarios where specific sections of the page could update dynamically using AJAX. For example, displaying course details based on the selected program or updating an application status indicator upon receiving an update from the server.
- Progress Bars: AJAX can be used to update progress bars during lengthy processes, like uploading application documents, providing visual feedback to the applicant.
Implementing AJAX:
Here’s a simplified breakdown:
- JavaScript Code: Write JavaScript code to handle user interactions (e.g., form submission, button click).
- Asynchronous Request: The JavaScript code sends an asynchronous request to a specific PHP script on the server using techniques like XMLHttpRequest.
- Server-side Processing: The PHP script processes the request, performs the necessary actions (e.g., validating data, retrieving data from the database), and prepares a response.
- Dynamic Update: The server sends a response (often in JSON format) back to the JavaScript code.
- JavaScript Updates UI: The JavaScript code receives the response and dynamically updates the web page content without a full page reload.
Remember: AJAX adds complexity to your codebase. Start with basic functionalities and gradually introduce AJAX for real-time updates where it enhances the user experience.
By implementing AJAX, you can transform your Admission System into a dynamic and user-friendly platform, improving the overall application experience for prospective students.
Reporting and Analytics:
Your Admission System isn’t just about collecting applications; it’s also about gleaning valuable insights from the data it gathers. Here, we’ll explore generating reports and utilizing analytics with PHP and MySQL to understand trends and make informed decisions.
- Leveraging MySQL Queries:
We can use MySQL queries to extract meaningful data from the database for reporting purposes. Here are some potential reports:
- Admission Statistics: Track the total number of applications received over time (e.g., monthly, yearly), the number of applications per course, or application completion rates.
- Applicant Demographics: Analyze applicant demographics like location, age range, or educational background to understand your applicant pool better.
- Trend Analysis: Identify trends in application volumes, program popularity, or application completion rates across different timeframes.
- Processing Data with PHP:
- You can use PHP to connect to the MySQL database and execute the desired queries to retrieve the necessary data.
- Process the retrieved data to calculate percentages, averages, or other relevant statistics.
- PHP can also be used to format the data for presentation in reports.
- Data Visualization with Libraries:
While raw data can be informative, visualizing it with charts and graphs significantly enhances its impact. Popular PHP libraries like Chart.js or Google Charts can be used to create visually appealing and easily digestible reports.
Benefits of Reporting and Analytics:
- Improved Decision Making: By analyzing trends and applicant demographics, you can make informed decisions about course offerings, admission requirements, or outreach strategies.
- Resource Allocation: Data can help you optimize resource allocation towards programs or demographics with higher application volume or success rates.
- Identify Challenges: Reports might reveal areas for improvement, such as low application rates for specific programs or high application abandonment rates at certain stages of the process.
Deployment and Testing
Deploying the Admission System to a web server and conducting thorough testing are crucial steps in ensuring the functionality, reliability, and security of the application. Let’s discuss the deployment process and the importance of comprehensive testing:
- Deployment Process:
- Choose a Web Hosting Provider: Select a web hosting provider that meets the requirements of the Admission System, including support for PHP, MySQL, and sufficient storage and bandwidth.
- Transfer Files to the Server: Upload the Admission System files to the web server using FTP (File Transfer Protocol) or a web-based file manager provided by the hosting provider.
- Configure Database Settings: Update the database connection settings in the application configuration files to point to the MySQL database hosted on the server.
- Set Permissions: Ensure that file and directory permissions are correctly set to allow the application to read, write, and execute files as needed.
- Test Deployment: Once the system is deployed, perform initial tests to ensure that it is accessible and functioning correctly on the live server.
- Importance of Thorough Testing:
- Functionality Testing: Test all features and functionalities of the Admission System to ensure they work as expected. This includes submitting admission applications, updating application status, and generating reports.
- Compatibility Testing: Test the system across different web browsers (e.g., Chrome, Firefox, Safari) and devices (e.g., desktop, tablet, mobile) to ensure compatibility and responsiveness.
- Security Testing: Conduct security testing to identify and mitigate potential vulnerabilities such as SQL injection, cross-site scripting (XSS), and session hijacking. Implement measures such as input validation, data sanitization, and secure authentication mechanisms.
- Performance Testing: Evaluate the performance of the system under various conditions, including peak traffic loads, to ensure scalability and responsiveness. Monitor server resources such as CPU usage, memory usage, and response times.
- User Acceptance Testing (UAT): Involve stakeholders, including administrators, staff, and prospective students, in user acceptance testing to gather feedback and ensure that the system meets their needs and expectations.
- Regression Testing: Perform regression testing to verify that recent changes or updates have not introduced any unintended side effects or broken existing functionality.
- Iterative Improvement:
- Continuous Monitoring: Monitor the live deployment of the Admission System to identify and address any issues or performance bottlenecks that may arise.
- Feedback Mechanism: Establish a feedback mechanism to gather feedback from users and stakeholders, allowing for continuous improvement and refinement of the system based on user experiences and needs.
- Regular Updates: Keep the Admission System up-to-date with security patches, bug fixes, and feature enhancements to ensure ongoing functionality, security, and usability.
In summary, deploying the Admission System to a web server and conducting thorough testing are essential steps in ensuring the system’s functionality, reliability, and security. By following best practices for deployment and testing, institutions can deploy a robust and secure Admission System that meets the needs of administrators, staff, and prospective students.
Conclusion
In this blog post, we have explored the development of an Admission System with Email Notification functionality using PHP. Let’s recap the key points discussed and emphasize the importance of such a system for educational institutions:
- Key Points Recap:
- We discussed the challenges faced by educational institutions in managing admissions, records, and communication with applicants.
- The benefits of using a web-based Admission System with Email Notification functionality were highlighted, including improved efficiency, data accuracy, and enhanced communication with applicants.
- We explored the implementation of various features and functionalities such as database design, user interface design, backend functionality, email notification, AJAX for dynamic updates, reporting, analytics, deployment, and testing.
- Importance of an Efficient Admission System with Email Notification:
- An efficient Admission System streamlines the admission process, reduces administrative overhead, and improves the overall user experience for both applicants and administrators.
- Email notification functionality ensures timely communication with applicants, keeping them informed about their admission status and providing additional instructions or requirements as needed.
- With an Admission System in place, educational institutions can better manage admissions, track application progress, analyze admission trends, and make data-driven decisions to improve their admission processes.
- Encouragement to Explore PHP’s Capabilities:
- PHP is a powerful and versatile programming language for web development, offering a wide range of features and functionalities for building dynamic web applications.
- Readers are encouraged to explore PHP’s capabilities further and consider developing their own Admission System or other web-based applications to streamline processes and enhance efficiency in their respective domains.
- With PHP’s extensive ecosystem of frameworks, libraries, and tools, developers have the resources they need to build robust and scalable web applications tailored to their specific requirements.
In conclusion, an efficient Admission System with Email Notification functionality is essential for educational institutions to manage admissions effectively, communicate with applicants seamlessly, and make informed decisions based on data insights. By leveraging PHP’s capabilities and following best practices in web development, institutions can deploy a modern and efficient Admission System that meets the needs of both administrators and applicants alike.
Credits to the developer of the project:
Readers are also interested in:
Cash loan and Pawning Monitoring System with SMS Using PHP
Email and SMS Marketing Solution in Laravel
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project