Salary Computation in CSharp
Introduction
Table of Contents
In the realm of payroll systems and financial applications, the calculation of one’s salary stands as a fundamental and recurrent task. Whether you are an employer looking to calculate your employees’ wages or an individual seeking to understand your own earnings, the ability to compute salaries accurately is indispensable. In this C# programming lesson, we embark on a journey to demystify the process of salary computation. Our goal is to create a C# console program capable of determining an individual’s salary based on key factors: the rate per day, the number of days worked, and deductions. By the end of this lesson, you will not only understand how to create such a program but also recognize the wider significance of salary computation in real-world financial scenarios.
The significance of salary computation extends across various industries and financial domains. From human resources departments managing payroll for large organizations to freelancers calculating their monthly earnings, the accurate determination of salaries ensures fairness, transparency, and financial stability. By delving into the world of salary computation, you are equipping yourself with a valuable skillset that holds relevance in both professional and personal financial management.
This lesson will guide you through the process of designing and building a C# console program for salary computation. We will start by defining the problem statement, identifying the necessary inputs, and formulating the salary calculation formula. You will learn how to collect user input, validate it for correctness, perform the salary calculation, and display the result effectively. By the end of this lesson, you will have a functional salary computation program in your coding arsenal, ready to tackle a variety of financial scenarios. So, let’s dive into the world of salary computation and empower ourselves with the tools to calculate earnings accurately.
Objectives
- Learn: Gain a comprehensive understanding of the principles behind salary computation, including the role of rate per day, number of days worked, and deductions in the calculation process. Grasp the fundamentals of C# programming required to create an effective salary computation program.
- Apply: Apply acquired knowledge to build a functional salary computation program in C#. Utilize the program to calculate salaries accurately for different scenarios, such as varying rates, work durations, and deduction amounts. Develop problem-solving skills by addressing potential input errors and validation challenges.
- Create: Independently design, develop, and customize a C# console program for salary computation. Create a user-friendly interface that collects necessary input data, performs calculations, and displays clear, well-formatted results. Experiment with various program features and functionalities to cater to diverse salary computation needs.
- Upgrade: Elevate your programming skills by enhancing the salary computation program. Explore opportunities to add advanced features, such as handling multiple employees or storing salary records. Implement error-handling mechanisms to improve program robustness. Continuously seek ways to optimize code efficiency and user experience, fostering a mindset of ongoing improvement.
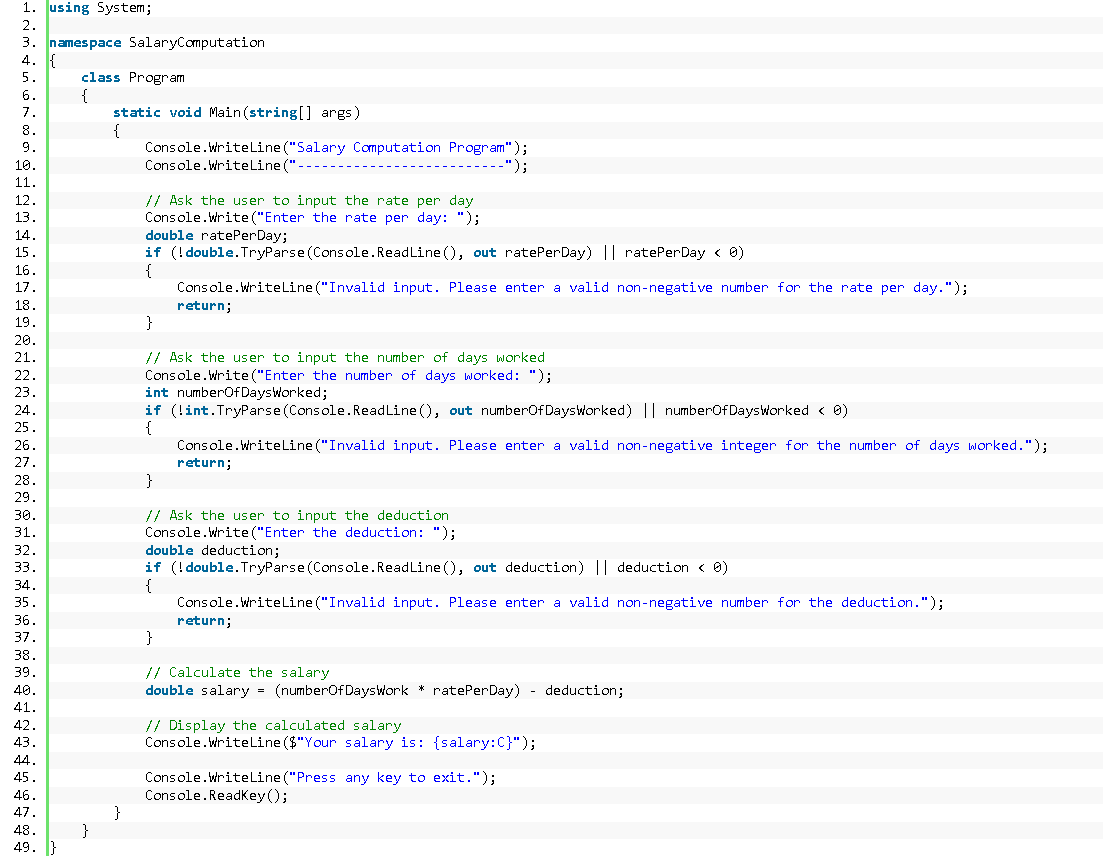
Source code example
using System; namespace SalaryComputation { class Program { static void Main(string[] args) { Console.WriteLine("Salary Computation Program"); Console.WriteLine("--------------------------"); // Ask the user to input the rate per day Console.Write("Enter the rate per day: "); double ratePerDay; if (!double.TryParse(Console.ReadLine(), out ratePerDay) || ratePerDay < 0) { Console.WriteLine("Invalid input. Please enter a valid non-negative number for the rate per day."); return; } // Ask the user to input the number of days worked Console.Write("Enter the number of days worked: "); int numberOfDaysWorked; if (!int.TryParse(Console.ReadLine(), out numberOfDaysWorked) || numberOfDaysWorked < 0) { Console.WriteLine("Invalid input. Please enter a valid non-negative integer for the number of days worked."); return; } // Ask the user to input the deduction Console.Write("Enter the deduction: "); double deduction; if (!double.TryParse(Console.ReadLine(), out deduction) || deduction < 0) { Console.WriteLine("Invalid input. Please enter a valid non-negative number for the deduction."); return; } // Calculate the salary double salary = (numberOfDaysWorked * ratePerDay) - deduction; // Display the calculated salary Console.WriteLine($"Your salary is: {salary:C}"); Console.WriteLine("Press any key to exit."); Console.ReadKey(); } } }
Explanation
This source code is for a C# console program that calculates an individual’s salary based on the rate per day, the number of days worked, and a deduction amount. Here’s a step-by-step explanation of the code:
- The program starts with a title and a separator to provide a clear visual indication of the purpose of the program.
- The program begins collecting input from the user:
- It first asks the user to input the rate per day using Console.Write(“Enter the rate per day: “). It then attempts to parse the user’s input as a double using double.TryParse(). If the input is not a valid non-negative number (ratePerDay < 0), it displays an error message and exits the program.
- Next, it asks the user to input the number of days worked and follows a similar process for validation.
- Finally, it asks for the deduction amount, validates it, and ensures it’s a valid non-negative number.
- After successfully collecting valid input for rate per day, number of days worked, and deduction, the program proceeds to calculate the salary:
- It multiplies the number of days worked (numberOfDaysWorked) by the rate per day (ratePerDay), which gives the earnings before deductions.
- It then subtracts the deduction amount (deduction) from the earnings to compute the final salary.
- The calculated salary is displayed using Console.WriteLine($”Your salary is: {salary:C}”), which formats the salary as currency using the “C” format specifier, making it more readable.
- Finally, the program waits for user input (Console.ReadKey()) before exiting. This allows the user to view the calculated salary before the program closes.
The code incorporates input validation to ensure that the user provides valid non-negative numbers for rate per day, days worked, and deduction. If any of the inputs are invalid, the program displays an error message and exits gracefully. Otherwise, it calculates the salary and presents the result to the user.
Output
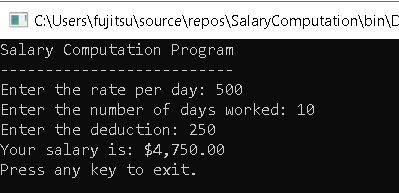
Summary
In this lesson, we explained how to create a C# console program for calculating a person’s salary. We broke down the process into simple steps, including input validation, salary calculation, and displaying the result. Key concepts covered include getting user input, checking if the input is valid numbers, performing math operations, and showing the result in a readable format.
Now that you’ve learned how to build this salary calculator, we encourage you to try it out and play around with the code. Don’t hesitate to make changes and explore different possibilities. You could consider adding features like handling taxes or accounting for overtime pay. By doing this, you’ll not only apply what you’ve learned but also improve your programming skills. Remember, practice is essential to becoming proficient in coding.
Armed with this knowledge, you’re ready to create practical programs for various purposes, such as payroll systems and financial tools. Keep coding, keep learning, and keep innovating. Your journey into the world of C# programming is just beginning!
Exercises and Assessment
Now that you’ve learned how to create a basic salary computation program in C#, it’s time to put your skills to the test and enhance the program’s functionality. Here are some challenges to help you improve your project:
- Tax Calculation: Modify the program to include tax calculations. Ask the user to input a tax rate, and then deduct taxes from the salary accordingly.
- Overtime Pay: Implement a feature that calculates overtime pay for hours worked beyond a standard workday (e.g., 8 hours a day). Ask the user to input the number of hours worked and the overtime rate, and then add the overtime pay to the salary.
- Multiple Employees: Extend the program to handle salary computations for multiple employees. Ask the user for the number of employees and loop through the process for each one, displaying individual salaries.
- Custom Deductions: Allow the user to specify different types of deductions (e.g., health insurance, retirement contributions) and input deduction amounts for each. Deduct these custom deductions from the salary.
Quiz
Let’s test your understanding of the Salary Computation Program’s source code example. Choose the correct answers for the following questions related to the provided code.
- What is the purpose of the “Salary Computation Program”?
a. To calculate the area of a circle
b. To compute a person’s salary
c. To convert currency
d. To find the average of numbers - In the program, what input is the user required to provide first?
a. Number of days worked
b. Deduction amount
c. Rate per day
d. Tax rate - What happens if the user enters a negative number for the rate per day?
a. The program terminates without an error message.
b. The program displays an error message and continues execution.
c. The program calculates the salary with the negative rate.
d. The program asks the user to re-enter the value. - Which of the following input validation checks is NOT performed in the program?
a. Checking if the number of days worked is an integer
b. Checking if the deduction amount is non-negative
c. Checking if the rate per day is a double
d. Checking if the user’s name is valid - What formula is used to calculate the salary in the program?
a. salary = rate per day + number of days worked – deduction
b. salary = (number of days worked * rate per day) – deduction
c. salary = (number of days worked + rate per day) * deduction
d. salary = (rate per day / number of days worked) – deduction - How is the calculated salary displayed to the user in the program?
a. As a plain number (e.g., 100)
b. As a percentage (e.g., 50%)
c. As currency (e.g., $100.00)
d. As scientific notation (e.g., 1.0E+2) - What action is taken after displaying the calculated salary to the user?
a. The program immediately exits.
b. The program asks the user for additional input.
c. The program waits for the user to press any key before exiting.
d. The program displays an error message. - If the user enters a valid but non-integer value for the number of days worked, what will happen?
a. The program displays an error message and continues execution.
b. The program calculates the salary with the non-integer value.
c. The program asks the user to re-enter the value as an integer.
d. The program terminates without an error message. - What is the primary purpose of the “Press any key to exit.” message at the end of the program?
a. To display the calculated salary again
b. To prompt the user for additional input
c. To notify the user that the program is complete
d. To display an error message - If the user enters a non-numeric value for any of the inputs, what action does the program take?
a. It converts the non-numeric value to zero.
b. It terminates without an error message.
c. It displays an error message and continues execution.
d. It calculates the salary based on the non-numeric value.
Related Topics and Articles:
Circle Properties Calculator in CSharp
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.