Circle Properties Calculator in CSharp
Introduction
Table of Contents
Welcome to the world of C# programming! In this lesson, we’re going to create a tool called the Circle Properties Calculator. But what does it do? Well, think of it as a helpful tool for finding out things about circles. You know, those round shapes? Specifically, it’ll figure out the diameter, area, and how long around the edge (circumference) a circle is. You tell it how big the circle is by giving it a number called the radius, and it’ll do all the math for you.
You might wonder why it’s useful to know stuff about circles. The truth is, circles are all around us, not just in textbooks. They show up in lots of real-life situations. For example, architects use circles when they design round parts of buildings or roads. Engineers need to get circle math right for things like making gears in machines. Even in everyday life, like figuring out how much pizza you have or how far you run around a circular track, understanding circle properties comes in handy. So, by the end of this lesson, you’ll not only have a nifty Circle Properties Calculator but also see how these circle calculations can help you in everyday situations. Let’s get started!
Objectives
Here are four outcomes-based objectives for the “Circle Properties Calculator in C#” lesson:
- Learn: By the end of this lesson, learners will have acquired a solid understanding of key mathematical concepts related to circles, including radius, diameter, area, and circumference.
- Apply: Students will be able to apply their knowledge to solve real-world problems that involve circular shapes, such as calculating the dimensions of circular objects or spaces.
- Create: Learners will demonstrate their programming skills by creating a functional Circle Properties Calculator program in C#, which can take user input and perform calculations to determine circle properties accurately.
- Upgrade: With a strong foundation in circle properties, students will have the skills and knowledge to enhance and expand the Circle Properties Calculator, adding additional features or improving its user interface for more advanced applications.
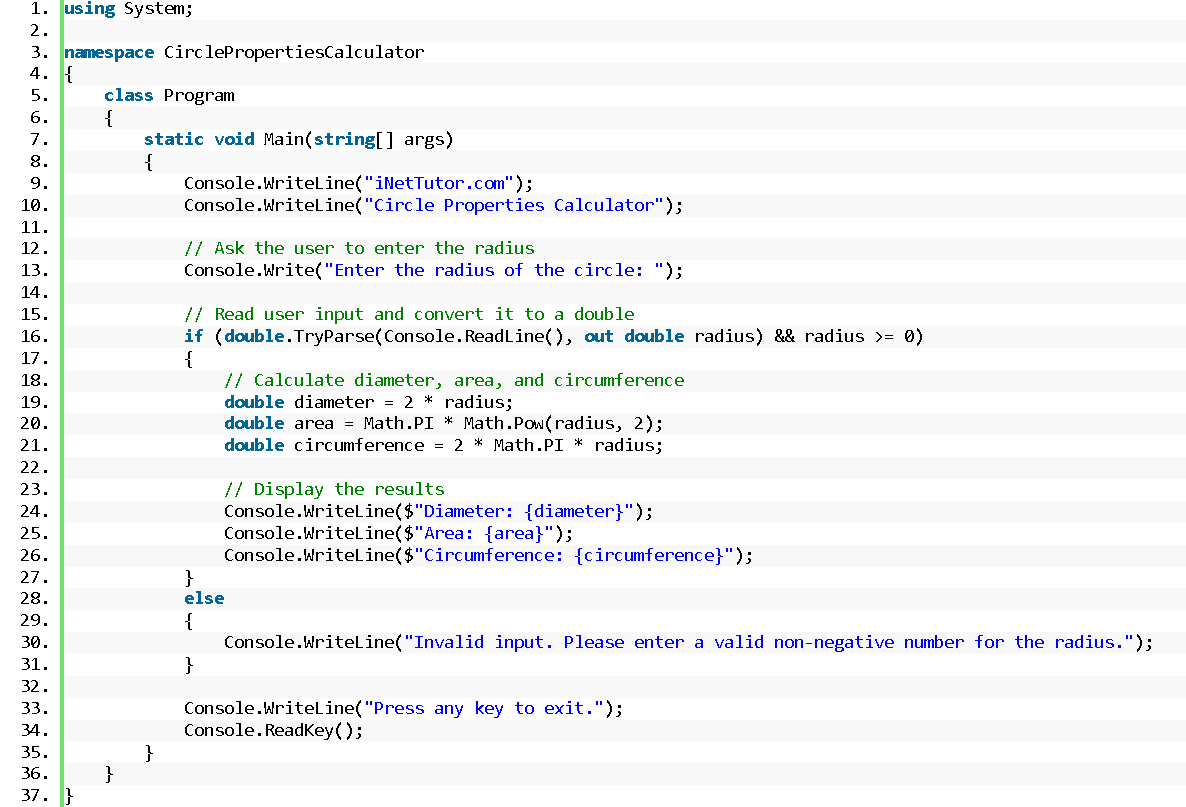
Source code example
using System; namespace CirclePropertiesCalculator { class Program { static void Main(string[] args) { Console.WriteLine("iNetTutor.com"); Console.WriteLine("Circle Properties Calculator"); // Ask the user to enter the radius Console.Write("Enter the radius of the circle: "); // Read user input and convert it to a double if (double.TryParse(Console.ReadLine(), out double radius) && radius >= 0) { // Calculate diameter, area, and circumference double diameter = 2 * radius; double area = Math.PI * Math.Pow(radius, 2); double circumference = 2 * Math.PI * radius; // Display the results Console.WriteLine($"Diameter: {diameter}"); Console.WriteLine($"Area: {area}"); Console.WriteLine($"Circumference: {circumference}"); } else { Console.WriteLine("Invalid input. Please enter a valid non-negative number for the radius."); } Console.WriteLine("Press any key to exit."); Console.ReadKey(); } } }
Explanation
This C# program is designed to calculate and display the properties of a circle based on user input, specifically the radius of the circle. Let’s break down the source code step by step:
- Using Statements:
- using System;: This statement includes the System namespace, which provides access to fundamental classes and functionality.
- Namespace:
- namespace CirclePropertiesCalculator: The program is organized within a namespace called CirclePropertiesCalculator, which helps in organizing related classes and program elements.
- Main Method:
- static void Main(string[] args): This is the entry point of the program, where execution begins when the program is run. It accepts an array of string arguments args, which are typically used for command-line input.
- Display Information:
- Console.WriteLine(“iNetTutor.com”): This line displays the program’s name or identifier, which is “iNetTutor.com.”
- Console.WriteLine(“Circle Properties Calculator”): This line displays a title indicating the purpose of the program, which is to calculate circle properties.
- User Input for Radius:
- Console.Write(“Enter the radius of the circle: “): This line prompts the user to enter the radius of the circle by displaying a message.
- if (double.TryParse(Console.ReadLine(), out double radius) && radius >= 0): It reads the user’s input as a string using Console.ReadLine(), tries to parse it as a double, and stores the result in the radius variable. The && radius >= 0 condition checks if the entered value is non-negative.
- Calculations:
- Inside the if block, calculations are performed based on the entered radius:
- double diameter = 2 * radius;: Calculates the diameter of the circle.
- double area = Math.PI * Math.Pow(radius, 2);: Computes the area of the circle using the formula πr².
- double circumference = 2 * Math.PI * radius;: Computes the circumference of the circle using the formula 2πr.
- Inside the if block, calculations are performed based on the entered radius:
- Display Results:
- Console.WriteLine($”Diameter: {diameter}”): Displays the calculated diameter.
- Console.WriteLine($”Area: {area}”): Displays the calculated area.
- Console.WriteLine($”Circumference: {circumference}”): Displays the calculated circumference.
- Invalid Input Handling:
- If the user enters invalid input (e.g., non-numeric or negative radius), the program will display the message: “Invalid input. Please enter a valid non-negative number for the radius.”
- Program Termination:
- Console.WriteLine(“Press any key to exit.”): Informs the user to press any key to exit the program.
- Console.ReadKey();: Waits for the user to press a key before the program exits.
Output
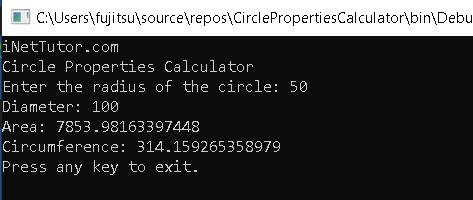
Summary
In this blog post, we embarked on a journey to demystify the process of creating a Circle Properties Calculator in C#. We learned how to create a simple yet powerful console application that calculates and displays the diameter, area, and circumference of a circle based on user input.
Key Concepts Recap:
- We explored the fundamental structure of a C# console application, including namespaces, the Main method, and the usage of the Console class for input and output operations.
- The program prompted the user to enter the radius of the circle, showcasing the interaction between the program and the user.
- We demonstrated the importance of input validation to ensure that the entered radius is a valid non-negative number.
- Calculations for the diameter, area, and circumference were carried out using basic mathematical formulas, demonstrating how C# can perform mathematical operations.
- The results were displayed in a clear and user-friendly format, enhancing the program’s readability and usefulness.
Encouragement to Experiment: I encourage you to take this code as a starting point for your C# programming journey. Experiment with it, modify it, and explore variations to gain a deeper understanding of C# and programming in general. Try different input validation techniques, formatting options, or even extend the program to calculate additional circle properties. Learning by doing is the best way to enhance your programming skills, so don’t hesitate to embark on your coding adventure with this Circle Properties Calculator!
In the world of programming, there are countless possibilities, and your creativity knows no bounds. Whether you’re a beginner or an experienced coder, the journey of exploration and experimentation is a valuable one. Happy coding!Top of Form
Exercises and Assessment
Exercises and Assessment for Improving the Circle Properties Calculator:
Exercise 1: Validate Radius
- Modify the program to provide more detailed error messages when invalid input is entered.
- Assess: Check if the program now provides specific error messages for different types of invalid input (negative numbers, non-numeric characters, etc.).
Exercise 2: User Interaction
- Enhance the user interaction by allowing users to input multiple circle radii and calculating properties for each one.
- Assess: Verify if the program can handle multiple radius calculations and display the results correctly.
Exercise 3: Additional Properties
- Extend the program to calculate additional circle properties, such as the area of a sector or the length of an arc based on user input.
- Assess: Ensure that the program correctly calculates and displays the additional circle properties.
Exercise 4: Error Handling
- Implement more robust error handling mechanisms, including catching exceptions and providing guidance to users on how to correct their input.
- Assess: Test the program with various invalid inputs and confirm that it gracefully handles exceptions and provides helpful guidance.
Assessment:
- Provide a set of test cases for the improved program, including valid and invalid inputs, and evaluate if the program produces correct results and handles errors gracefully.
- Assess the program’s overall code quality, readability, and adherence to best practices.
- Encourage learners to explain the improvements they made and their reasoning behind them.
Quiz
- What is the main purpose of the “Circle Properties Calculator” program?
a) Calculate the volume of a sphere
b) Compute the properties of a circle based on user input
c) Convert units of length
d) Perform complex mathematical calculations - Which circle properties are calculated by the program?
a) Area and perimeter
b) Diameter, area, and circumference
c) Radius and area
d) Circumference and radius - In the code, what does Math.PI represent?
a) The radius of the circle
b) The mathematical constant π (pi)
c) The diameter of the circle
d) The circumference of the circle - How is the user’s input for the radius validated in the program?
a) Using regular expressions
b) By checking if it’s an integer
c) By ensuring it’s a positive number
d) By comparing it to a predefined value - What does the program do if the user enters an invalid input for the radius?
a) Terminates the program
b) Displays an error message and continues
c) Ignores the input and proceeds with calculations
d) Prompts the user to re-enter the radius - How is the diameter of the circle calculated in the program?
a) By multiplying the radius by 2
b) By dividing the circumference by 2
c) By taking the square root of the radius
d) By squaring the radius - Which C# data type is used to store the radius of the circle?
a) string
b) double
c) int
d) char - What message does the program display before asking the user to enter the radius?
a) “Please enter the diameter of the circle:”
b) “Enter your name:”
c) “Enter the radius of the circle:”
d) “Choose an operation (add/subtract/multiply/divide):” - What will be displayed as the program output if the user enters a radius of 5.0?
a) Diameter: 10.0 Area: 78.54 Circumference: 31.42
b) Diameter: 5.0 Area: 25.0 Circumference: 15.7
c) Diameter: 2.5 Area: 19.63 Circumference: 15.7
d) Diameter: 5.0 Area: 78.54 Circumference: 78.54 - What action does the program take after displaying the results?
a) It prompts the user to choose another operation.
b) It waits for the user to press any key before exiting.
c) It clears the console screen.
d) It automatically terminates without waiting for user input.
Related Topics and Articles:
Calculate Average of Numbers in CSharp
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.