Calculate Average of Numbers in CSharp
Introduction
In this lesson, we’ll create a C# program with a simple yet valuable purpose: calculating the average of numbers entered by the user. This seemingly straightforward task holds great significance across a multitude of domains. Whether you’re in the realm of statistics, finance, or even game development, understanding how to compute averages is a fundamental skill that forms the basis of data analysis. By the end of this lesson, you’ll be equipped with the knowledge and tools to create your own average calculator in C #and you’ll have a solid grasp of the underlying concepts.
Why is calculating averages such an essential skill? Averages offer us a quick and concise way to summarize data. Think of them as a snapshot of the “typical” value within a dataset. In statistics, averages are used to analyze survey results, track trends, and make informed decisions. In finance, they help assess financial performance. Even in everyday life, calculating averages is handy for determining things like your monthly expenses or the average temperature over a week. Averages simplify complex data, making it easier to draw meaningful insights and predictions.
Let’s take a peek at what lies ahead in this lesson:
- Prerequisites: We’ll start by briefly mentioning what you need to get started, including a basic understanding of C# and the development environment.
- Program Structure: You’ll learn about the fundamental structure of a C# program, including the role of namespaces, classes, and the Main method.
- User Input and Looping: We’ll explore how to prompt the user for input and create a loop to handle multiple numbers.
- Calculating the Average: We’ll delve into the core logic of accumulating numbers and calculating the average.
- Outputting the Result: You’ll discover how to present the calculated average in a user-friendly way.
- Conclusion: We’ll wrap things up with a recap of key concepts, encouragement to experiment, and pointers for further learning.
So, fasten your seatbelt as we embark on this enlightening journey into the world of C# programming and the power of calculating averages!
Objectives
- Learn: By the end of this lesson, you will have acquired a comprehensive understanding of the C# programming language’s fundamental concepts, including data types, variables, and basic syntax.
- Apply: You will be able to apply your knowledge to write simple C# programs, including those that perform calculations, handle user input, and display results.
- Create: You will have the skills to create a C# program that calculates the average of numbers entered by the user, demonstrating your ability to design, develop, and implement practical applications.
- Upgrade: Building on your newfound knowledge, you’ll have the foundation to tackle more complex programming challenges, explore advanced C# features, and continue your journey to becoming a proficient C# developer.
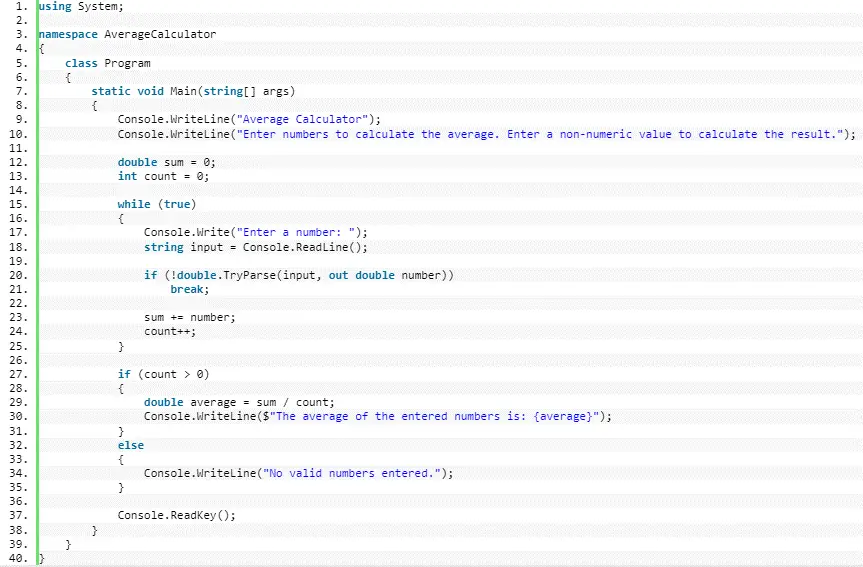
Example Source code
using System; namespace AverageCalculator { class Program { static void Main(string[] args) { Console.WriteLine("Average Calculator"); Console.WriteLine("Enter numbers to calculate the average. Enter a non-numeric value to calculate the result."); double sum = 0; int count = 0; while (true) { Console.Write("Enter a number: "); string input = Console.ReadLine(); if (!double.TryParse(input, out double number)) break; sum += number; count++; } if (count > 0) { double average = sum / count; Console.WriteLine($"The average of the entered numbers is: {average}"); } else { Console.WriteLine("No valid numbers entered."); } Console.ReadKey(); } } }
Explanation
Here’s a breakdown of how the program works:
- We start by displaying a welcome message and an explanation of what the program does.
- We declare two variables, sum to store the sum of the entered numbers, and count to keep track of how many numbers have been entered.
- We use a while loop to repeatedly ask the user for input until they enter a non-numeric value (a value that cannot be parsed as a double).
- Inside the loop, we read the user’s input as a string and attempt to parse it as a double. If parsing succeeds, we add the number to the sum and increment the count.
- When the user enters a non-numeric value, the loop exits.
- We check if any valid numbers were entered (i.e., count is greater than 0). If so, we calculate the average by dividing the sum by the count and display it.
- If no valid numbers were entered, we display a message indicating that no valid numbers were provided.
- Finally, we use Console.ReadKey() to wait for the user to press a key before the program exits.
You can run this program in a C# development environment, and it will allow you to enter numbers and calculate their average.
Output
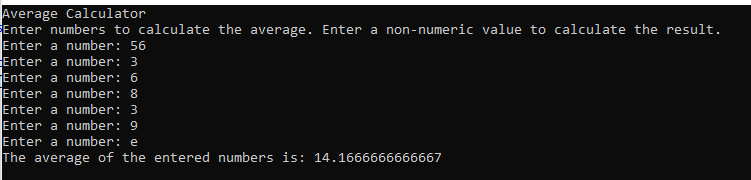
Summary
In this lesson, we explored into essential concepts such as data types, variables, user input, arithmetic operations, and conditional statements. We explained the syntax of C# code and learned how to design a program that calculates the average of numbers entered by the user. Understanding these core principles sets a strong foundation for your journey into C# programming.
Encouragement to Experiment: Now that you’ve grasped the basics, it’s time to embark on your own coding adventures. Don’t hesitate to experiment with the program you’ve created—try modifying it to calculate other statistical values, implement error handling, or enhance the user interface. The best way to solidify your learning is through hands-on experience, so keep coding, keep exploring, and watch your C# skills grow. Happy coding!
Assessment
To enhance the program that calculates the average of numbers entered by the user in C# console, you can consider the following assessment tasks:
Error Handling: Add error handling mechanisms to handle scenarios where the user enters non-numeric values or attempts to divide by zero. Provide meaningful error messages and guide the user to correct their input.
Validation: Implement input validation to ensure that the user enters a minimum number of values before calculating the average. For example, you could require the user to enter at least two numbers.
Enhanced Calculation: Go beyond calculating just the average. Offer options to calculate other statistical values, such as the sum, minimum, maximum, or standard deviation of the entered numbers.
Quiz
1. In the C# program, what is the primary purpose of the program?
A. Calculate the sum of entered numbers.
B. Calculate the average of entered numbers.
C. Calculate the product of entered numbers.
D. Calculate the maximum of entered numbers.
2. Which part of the program is responsible for handling user input?
A. The Console.WriteLine statements.
B. The if statement.
C. The Convert.ToDouble(Console.ReadLine()) expression.
D. The Console.WriteLine(“Average: ” + average); statement.
3. How does the program handle non-numeric input from the user?
A. It converts non-numeric input to zero.
B. It displays an error message and asks the user to re-enter the input.
C. It ignores non-numeric input.
D. It terminates the program.
4. What is the purpose of the total variable in the program?
A. To store the sum of all entered numbers.
B. To count the number of numbers entered.
C. To store the maximum of all entered numbers.
D. To store the average of all entered numbers.
5. Which part of the program calculates the average of the entered numbers?
A. average = total;
B. average = total / count;
C. average = count / total;
D. average = total * count;
6. If a user enters three numbers: 10, 20, and 30, what will be the calculated average?
A. 10
B. 20
C. 60
D. 15
7. What type of loop is used in the program to continuously accept user input until a specific condition is met?
A. for loop
B. while loop
C. do-while loop
D. switch loop
8. What will happen if the user enters no numbers and presses Enter immediately?
A. The program will crash.
B. The program will display an error message.
C. The program will calculate an average of zero.
D. The program will wait indefinitely for input.
Related Topics and Articles:
Currency Converter Program in CSharp
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.