Read and Update Text File Content in VB.NET
Problem
Create a Windows Form Application program in Visual Basic.Net that will allow users to read a text file and update its contents.
Description
This tutorial will allow the user to read a text file and allows updating it using VB.NET.
Before the tutorial the following are required to start:
- Microsoft Visual Studio 2008 – Above
The tutorial starts here:
- Open Microsoft Visual Studio 2012
- Select a New Project on the File menu.
- Select Visual Basic, Windows Form Application then click OK.
- We need to design our form by the following controls:
- 2 Button – 1 button to Read the file and 1 button to update the content
- 1 Text box – text box for the Text.
- We will also name our form controls in this way:
- txttext is the name of the textbox.
- cmdread is the name of the button for the read button.
- cmdupdate is the name of the button for the update button.
- This is how we design the form. (Feel free to layout your own)
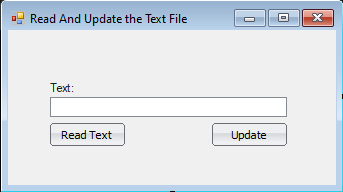
Figure 1. Design of the Form
- Import the following by pasting the code at the top of the text editor.
Code here
Imports System.Runtime.InteropServices Imports System.Runtime.CompilerServices Imports System.IO Imports System.Text
End Code
Code Explanation:
It imports some system services that could read and update the file.
- Paste the code below to add a Public Function.
Code here
Public Function GetSettingItem(ByVal File As String, ByVal Identifier As String) As String On Error GoTo MyError Dim S As New IO.StreamReader(File) : Dim Result As String = "" Do While (S.Peek <> -1) Dim Line As String = S.ReadLine If Line.ToLower.StartsWith(Identifier.ToLower & "=") Then Result = Line.Substring(Identifier.Length + 1) End If Loop : S.Close() Return Result : S.Close() MyError: End Function <DllImport("kernel32")> Private Shared Function WritePrivateProfileString(ByVal lpSectionName As String, ByVal lpKeyName As String, ByVal lpString As String, ByVal lpFileName As String) As Long End Function Public Event Datareceived(ByVal sender As Object, ByVal Data As String)
End Code
Code Explanation:
This code will serve as the reader of the text file. Also, a kernel32.dll was imported in VB.NET and a Public Event was added to fully read the text.
- Paste the code below to add a Private function.
Code here
Private Function SetIniValue(section As String, key As String, filename As String, Optional defaultValue As String = "") As String Dim sb As New StringBuilder(500) If WritePrivateProfileString(section, key, defaultValue, filename) > 0 Then Return sb.ToString Else Return defaultValue End If End Function
End Code
Code Explanation:
This code will help allow the user to update or overwrite the text file.
- double click the read button and paste the following
Code here
txttext.Text = GetSettingItem(Application.StartupPath & "/readme.ini", "readme")
End Code
Code Explanation:
This code will get the text file and read the contents
- double click the update button and paste the following
Code here
SetIniValue("text", "readme", Application.StartupPath & "/readme.ini", txttext.Text) txttext.Text = ""
End Code
Code Explanation:
This code will update the text file and clears the text box.
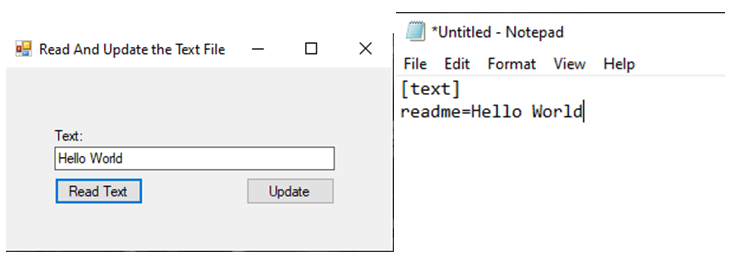
Author:
Name: Charlie Devera
Email Address: charliedevera000@gmail.com
Free Download Source code (Read and Update Text File Content in VB.NET)
You may visit our facebook page for more information, inquiries and comments.
Hire our team to do the project.