In the previous lesson we have enumerated the different data types in visual basic, now we’re going to use those data types in a real scenario. In this lesson, we will about to learn declaring variables with its corresponding data type.
Let us first define what a variable is.
In computer programming, a variable is something that can hold a piece of data or value. Every variable must have a name (variable name) and every variable must have its data type such as integer, Boolean, string, etc.
In visual basic you can assign a constant value in your variable and other variable may change its value during the execution of the program.
Declaring Variable
In visual basic, declaration of variable starts with the Dim statement
Dim myName as String
Dim myAge as Integer
In the above example we have declared two variables namely myName and myAge, myName is a string type in which it can accept alpha-numeric value whereas myAge is an integer type that can only store numbers.
Naming convention
- A variable must not be a reserved word
- A variable must be readable
- A variable must imply its data type
- A variable must start with a letter (upper case or lower case). No spaces and punctuation marks are allowed
- A variable can be as long as 255 characters.
Note: in visual basic variables are not case sensitive (myAge, myage, MyAge) they are all the same. But as a rule of thumb it is recommended to make your variable consistent and properly formatted.
Assigning value to a variable
A variable is useless without anything inside it. So let’s put some value on our variable.
The main purpose of a variable is to hold a temporary or constant value. We will give example on those two values (static and dynamic value).
Static value
Earlier we have stated that a variable can contain a constant value and other may change during the execution of the program.
Here’s how to assign a constant value to a variable
Private Sub Form_Load()
Dim myName As String
Dim myAge As Integer
myName = "Jose"
myAge = 19
MsgBox myName & " " & myAge
End Sub
Note: this method is not flexible because you can’t change the value during runtime, unless you’re going to modify the source code.
This example will demonstrate how to assign a value to our variable via textbox control in visual basic.
Private Sub Command1_Click()
Dim myName As String
Dim myAge As Integer
myName = Text1.Text
myAge = Text2.Text
MsgBox myName & " " & myAge
End Sub
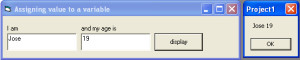
In the example above the value of our variable will depend on the input of the user in the text field which makes this method more dynamic because we don’t need to modify the source code just to change the value.
During runtime, myName variable will get its value from Text1 and myAge variable will get its value from Text2, when the user clicks the display button message box will pop-up the display the value entered by the user.