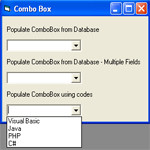
Populate Combo Box from database in Visual Basic 6
Combo Box in Visual Basic also refers as drop down lists or drop down boxes.
In this tutorial, we will going to learn two ways on how to add or populate item in the combo box.
Two ways to add or populate item in our combo box:
1. Via database record
2. Via manual coding
Populate combo box from database
Designing the Database and Table
1. We must first create a MS Access database and name it as Data.mdb.
2. Create a new table and name it as tblStudent.
3. Save the table and insert the following field:
Field name – Data Type
ID – Autonumber
studentName – Text
studentCountry– Text
The table should look like the image below:
4. Insert records in our newly created table. Fill in only the studentName and studentCountry since ID is AutonNumber it will automatically assign a number to the record.
5. Close the table.
After we have created our database, let us proceed in our visual basic program.
We will skip the procedures on how to connect visual basic 6 to ms access, but if you forgot that, kindly visit the tutorial on how to connect vb6 to ms access.
1. Open your visual basic 6 program and create a new project, select Standard EXE.
2. Let’s add a form to our project and name it as ComboBoxFrm.
3. In the Tool Box of our Visual Basic 6 program, kindly drag the combo box control to our newly created form (ComboBoxFrm).
In the image below, the highlighted one is our ComboBox control.
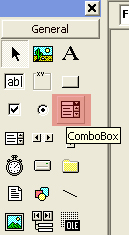
4. Rename the combo box into cmbDatabaseCombo.
5. Let’s create a function that will retrieve the records from our table and pass it to our combo box.
Copy the code below and paste in our ComboBoxFrm form:
Public Sub AddStudentName()
Dim sql As String
If rs.State = adStateOpen Then rs.Close
sql = " Select studentName from tblStudent Group by studentName order by studentName"
rs.Open sql, conn
cmbDatabaseCombo.Clear
Do While Not rs.EOF
cmbDatabaseCombo.AddItem rs!studentName
rs.MoveNext
Loop
End Sub
The query above will get all the records under studentName and pass it over to our combo box (cmbDatabaseCombo).
6. The code above will only execute its function once it we will call it. To call a function or subroutine in Visual Basic, just type the name of that function or Sub procedure. In our case we will put the function in the load event of the form.
Double click the ComboBoxFrm form and type the Sub procedure (AddStudentName). The code will look like this:
Private Sub Form_Load()
AddStudentName
End Sub
7. Test the project by pressing F5. The output of the project will be like the image below.
Multiple Fields
We can also combine to two or more fields from our database and pass it over to our combo box.
Here’s the sample code:
Public Sub AddMultipleField()
Dim sql As String
If rs.State = adStateOpen Then rs.Close
sql = " Select studentName,studentCountry from tblStudent Group by studentName,studentCountry order by studentName"
rs.Open sql, conn
cmbDatabaseComboMultiple.Clear
Do While Not rs.EOF
cmbDatabaseComboMultiple.AddItem rs!studentName & " - " & rs!studentCountry
rs.MoveNext
Loop
End Sub
Add this Sub procedure (AddMultipleField) in the load event of our form.
The output should look like this:
The manual procedure of adding items into combo box
The code below is a simple way of adding items into our combo box:
Public Sub AddManual()
cmbCodes.Clear
cmbCodes.AddItem ("Visual Basic")
cmbCodes.AddItem ("Java")
cmbCodes.AddItem ("PHP")
cmbCodes.AddItem ("C#")
End Sub
Add this Sub procedure (AddManual) in the load event of our form.
The output of the above code will be like this:
We have now added three Sub procedures in the load event of our form:
Private Sub Form_Load()
AddStudentName
AddMultipleField
AddManual
End Sub
The source code of this tutorial is available for download.
This is the end of our tutorial. Hope you have learned something from this.
Feel free to post your comments and suggestions.
autocomplete text Box from database in Visual Basic 6. i want 3 coloumn