Dynamic Drop down in PHP and MySQL Free Source code and Tutorial
Introduction
Table of Contents
A drop-down list is a graphical control element, similar to a list box, that allows the user to choose one value from a list. When a drop-down list is inactive, it displays a single value. When activated, it displays a list of values, from which the user may select one. When the user selects a value, the list is hidden and the value is displayed in the text field beneath. A combo box is a control element that allows the user to select one or more values from a list, grid, or table. When activated, it displays a collection of drop-downs (or radio buttons), each of which has one or more possible values. When the user selects a value, the combo box is hidden and the selected value is displayed in the text field beneath.
There are two parts to a drop-down list in PHP and MySQL: the HTML code that defines the list, and the PHP code that fetches the data from the MySQL database. This article will guide you through the development of creating a dynamic dropdown in PHP and MySQL. Let’s get started with the development.
Objectives
By the end of this tutorial, you will be able to:
- Create a PHP script that connects to MySQL database.
- Fetch the record from our database table.
- Create a front-end in Bootstrap where the data fetched in the table will be displayed in the drop down menu.
- To integrate and apply the source code in your projects.
Relevant Source code
You’ll need a basic understanding of both PHP and MySQL to write a tutorial on exporting data using PHP and MySQL. You’ll also need access to a server that can run PHP and MySQL. Once you have these items, you may create your tutorial by following the instructions below.
- XAMPP
- Text editor (VS Code, Sublime, Brackets), download and install a text editor of your choice
- Let us start first by creating our database. In the download file, you can just import the city.sql file in you PHPMyAdmin or you can just copy paste the create table statement below.
city.sql
-- Table structure for table `tblcity` CREATE TABLE `tblcity` ( `id` int(11) NOT NULL, `city_name` varchar(50) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4; -- Dumping data for table `tblcity` INSERT INTO `tblcity` (`id`, `city_name`) VALUES (1, 'Pasig'), (2, 'Quezon'), (3, 'Makati'), (4, 'Taguig'); -- Indexes for table `tblcity` ALTER TABLE `tblcity` ADD PRIMARY KEY (`id`);
- We will now establish the connection to our MySQL database.
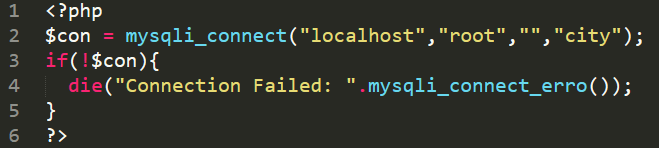
<?php $con = mysqli_connect("localhost","root","","city"); if(!$con){ die("Connection Failed:". mysqli_connect_error()); } ?>
Line 1-6 – first we need to connect to our mysql database server. For XAMPP, the default host is localhost, database username is root, there is no password and the name of the database is city.
- Lastly, we will now create the form and PHP script that will fetch the data from our database table.
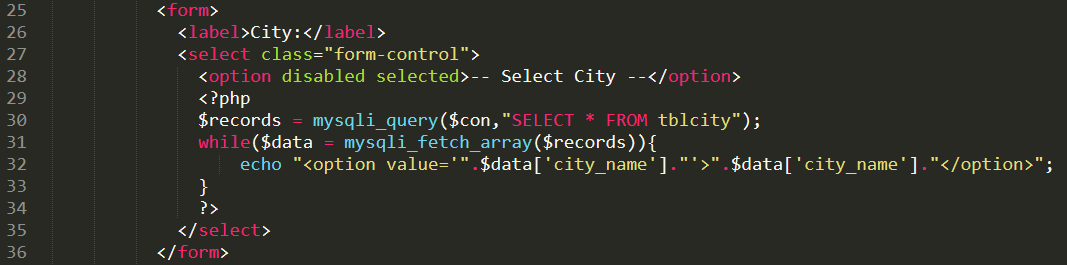
<form> <label>City:</label> <select class="form-control"> <option disabled selected>-- Select City --</option> <?php $records = mysqli_query($con,"SELECT * FROM tblcity"); while($data = mysqli_fetch_array($records)){ echo "<option value='".$data['city_name']."'>".$data['city_name']."</option>"; } ?> </select> </form>
Line 25-36 – this is the form where the drop down is located. It also includes the PHP script that will fetch the data from our database table and populate our drop down. The name of the database table is tblcity and it has two columns the id and city_name.
Video Demo
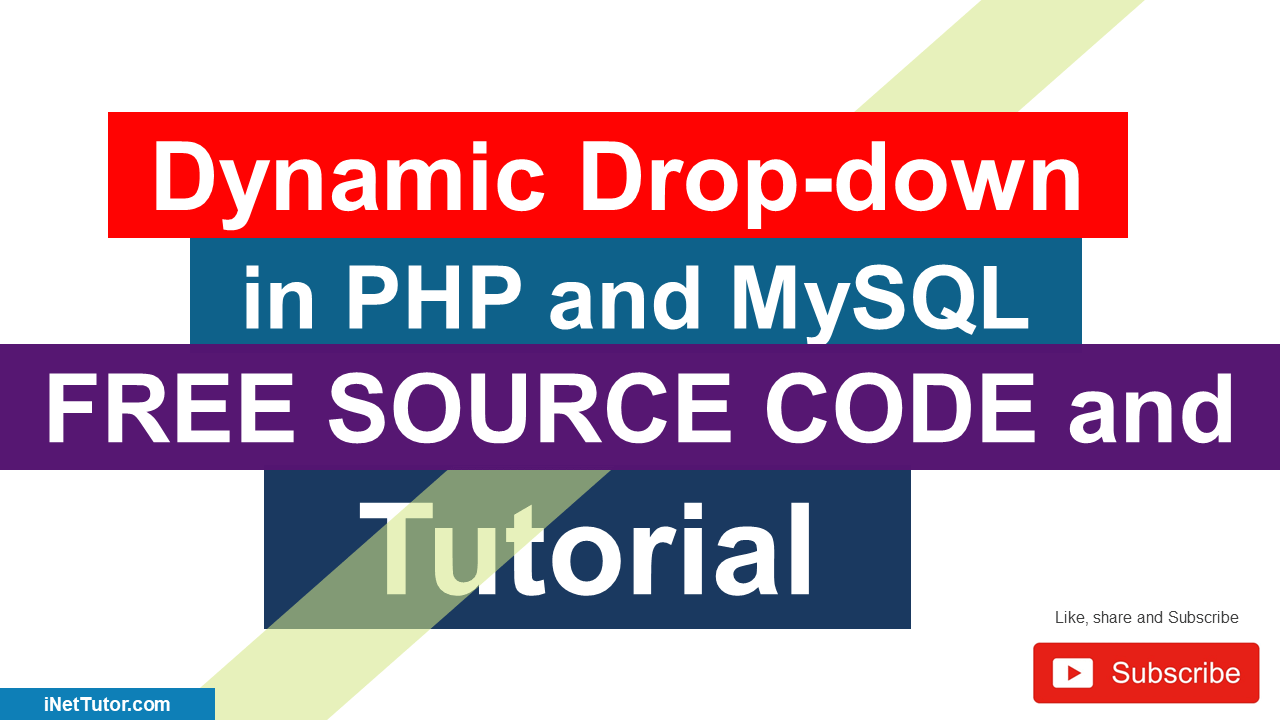
Summary
A drop down menu is an important part of any website that uses PHP and MySQL. It allows the user to select an option from a list of options, without having to type anything in. This can be very useful for long lists of options, or for lists that are constantly changing. Drop down menus can also be used to submit forms, or to display information about a particular category of items. By using a drop down menu, you can make your website much more user-friendly. With this tutorial, we can create a feature that allows us to add, modify or even delete the list from our drop down without changing the actual HTML code.
In this article, you can download the source code and as well as the database used in creating the tutorial on creating a dynamic drop down in PHP and MySQL.
We hope you found this tutorial to be helpful! Wishing you the best of luck with your projects! Happy Coding!
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.
Related Topics and Articles: