Generate Random Number in PHP
Introduction
There are many ways to generate a random number in PHP. The most common way is to use the rand() function. This function takes two parameters – a minimum value and a maximum value. The function will return a random number between the two values. In this tutorial we will be creating a PHP script that will ask the user to enter two numbers and randomly display a number between the min value and max value.
Objectives
By the end of this tutorial, you will be able to:
- Create a front-end form in Bootstrap that allows the user to enter a min number and max number.
- To create a PHP script that process the user input and display a random number using the rand() function.
- To integrate and apply the source code in your projects.
You may also want to read: Best PHP PDF Tutorials for Beginners
Source code explanation
Requirements
- XAMPP
- Text editor (VS Code, Sublime, Brackets), download and install a text editor of your choice
1. The first thing to do is to create a form that will capture the user input and submit it for the processing in the PHP script. We need two text boxes; 1 for the minimum value, and 1 for the maximum value or number. In this form, we need also a button that will submit the values entered to our back-end.

<form method="post"> Min: <input type="number" name="min" min="0" value="1" class="form-control"><br> Max: <input type="number" name="max" min="1" value="1000" class="form-control"><br> <input type="submit" name="submit" class="btn btn-primary" value="Generate"> </form>
2. Next is to prepare our PHP script that will process the user input.

<?php if(isset($_POST['submit'])){ $min = $_POST['min']; $max = $_POST['max']; $rand_num = rand($min,$max); echo "<h4>Random Number between ".$min. " and ".$max." is ".$rand_num."</h4>"; } ?>
Line 25-32 – this is the scope of the PHP script that will process the user input and display a random number between the minimum value and maximum value.
Line 27 – we have a variable $min that will hold the minimum value entered in the form.
Line 28 – $max variable is to store the max value entered by the user in the form.
Line 29 – in this line, we have created a variable that will store the random number using the rand() function.
To generate a random integer, use the rand() function. It has two parameter; min and max
min: The lowest value to be returned is specified by this optional argument. 0 is the default value.
max: It defines the highest value to be returned and is an optional argument.
Generate Random Number in PHP PDF Tutorial
Output
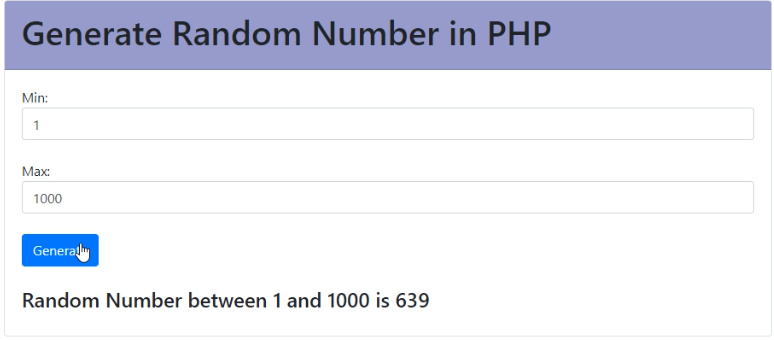
Complete Source code
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0, shrink-to-fit=no"> <title>Generate Random Number in PHP</title> <link rel="stylesheet" href="assets/bootstrap/css/bootstrap.min.css"> </head> <body> <div class="row"> <div class="col-md-8 offset-md-2"> <div class="card"> <div class="card-header bg"> <h1>Generate Random Number in PHP</h1> </div> <div class="card-body"> <form method="post"> Min: <input type="number" name="min" min="0" value="1" class="form-control"><br> Max: <input type="number" name="max" min="1" value="1000" class="form-control"><br> <input type="submit" name="submit" class="btn btn-primary" value="Generate"> </form> <br> <?php if(isset($_POST['submit'])){ $min = $_POST['min']; $max = $_POST['max']; $rand_num = rand($min,$max); echo "<h4>Random Number between ".$min. " and ".$max." is ".$rand_num."</h4>"; } ?> </div> </div> </div> </div> <script src="assets/js/jquery.min.js"></script> <script src="assets/bootstrap/js/bootstrap.min.js"></script> </body> </html>
Summary
In this tutorial, we have created a PHP script that will display a random number between the minimum value and maximum value. To make it more interactive, we have also created a form to capture the user input and pass the value into our variables that will allow the PHP script to process.
Numerous applications for random numbers exist, ranging from producing random results in games to producing random samples for statistical research. In order to ensure that each participant has an equal probability of receiving any given treatment, they can also be used to randomize trials. To prevent messages from being read by unwanted parties, cryptography also uses random numbers. In some cases, the random number generator can also be used for CAPTCHA that ensure that the response is generated by a human being. The random number generator is necessary in each of these instances to guarantee that the outcomes are fair and unpredictable. In addition to being a useful tool in many real-world applications, random numbers can be a fascinating method to study many mathematical ideas.
We hope you found this tutorial to be helpful! Wishing you the best of luck with your projects! Happy Coding!
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.